Access Methods and Efficiency Comparison of unordered_map Elements
发布时间: 2024-09-15 18:20:18 阅读量: 27 订阅数: 22 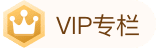
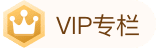
# 1. Introduction to unordered_map
The unordered_map is an associative container in the C++ ***pared to the map, the unordered_map does not require elements to be stored in any specific order but uses a hash function to quickly locate elements. This feature makes the unordered_map more efficient in operations like insertion, lookup, and deletion, particularly suited for processing large volumes of data. Part of the STL, it allows for operations such as insertion, deletion, and lookup using standard container operations, making programming more convenient.
The unordered_map ensures the uniqueness of key-value pairs, meaning the same key can only correspond to one value. Introduced after C++11, it offers developers more options for data structures, greatly facilitating applications in various scenarios. The use cases for unordered_map are broad, particularly suitable for tasks that require efficient data lookup and insertion.
# 2. Using unordered_map
The unordered_map container offers a simple and efficient way to store key-value pairs. Below we will delve into how to perform insertion, deletion, and lookup operations using unordered_map.
### 2.1 Inserting Elements
There are two common methods for inserting elements into an unordered_map: using the `insert` function and using the `emplace` function.
#### 2.1.1 Using the insert Function
The `insert` function can add a key-value pair to the unordered_map. If the key already exists, no insertion will occur.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> umap;
// Insert elements using the insert function
umap.insert(std::make_pair(1, "apple"));
umap.insert(std::make_pair(2, "banana"));
return 0;
}
```
#### 2.1.2 Using the emplace Function
The `emplace` function is similar to the `insert` function but constructs elements directly at the parameter position, offering higher efficiency.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> umap;
// Insert elements using the emplace function
umap.emplace(1, "apple");
umap.emplace(2, "banana");
return 0;
}
```
### 2.2 Deleting Elements
The unordered_map also provides methods for removing elements, including the `erase` function for deleting elements with a specific key or the `clear` function to empty the entire container.
#### 2.2.1 Using the erase Function
The `erase` function can remove elements with a specified key.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> umap = {{1, "apple"}, {2, "banana"}};
// Delete elements using the erase function
umap.erase(1);
return 0;
}
```
#### 2.2.2 Using the clear Function
The `clear` function can empty the entire unordered_map container.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> umap = {{1, "apple"}, {2, "banana"}};
// Clear the container using the clear function
umap.clear();
return 0;
}
```
# ***paring unordered_map with Other Containers
As one of the associative containers in the standard library, unordered_map has unique characteristics and use cases compared to other containers. In the following content, we will compare unordered_ma
0
0
相关推荐
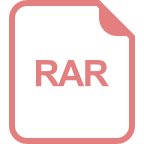
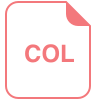
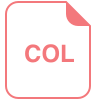
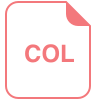
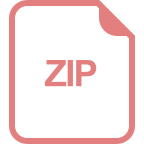
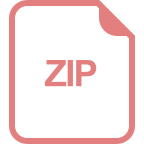
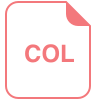