Concurrent Operations and Lock Mechanisms on Unordered Maps
发布时间: 2024-09-15 18:30:56 阅读量: 7 订阅数: 15 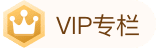
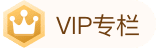
# Introduction
In programming, unlocked operations can lead to data races and unpredictable outcomes, causing program crashes or abnormal behavior. Concurrent operations refer to the situation where multiple threads or processes access shared resources at the same time, and special attention is needed when performing concurrent operations on unordered_map. Unordered_map is a hash table container provided by C++ STL, which has fast lookup and insertion performance. In concurrent programming, it is necessary to understand the basic concepts and challenges of concurrent operations, as well as the usage and precautions of locking mechanisms. This article will introduce the basic concepts of unordered_map, the basics of concurrent programming, and how to perform concurrent operations on unordered_map, helping readers better understand the tips for using unordered_map in concurrent programming.
# Basic Concepts of unordered_map
Unordered_map, or unordered hash table, is an associative container in C++ STL. Unlike map, the elements in unordered_map are stored in an unordered manner, and the fast lookup is achieved through a hash table. In unordered_map, each element is a key-value pair, and the relationship between key and value is one-to-one. Unordered_map provides fast lookup, insertion, and deletion operations, making it suitable for scenarios where fast element lookup is required.
#### Introduction to unordered_map
Unordered_map is defined in the `<unordered_map>` header file, with the syntax `std::unordered_map<key_type, value_type>`. Here, `key_type` represents the type of the key, and `value_type` represents the type of the value. Unordered_map uses a hash table to store data, allowing it to complete lookup operations in O(1) time.
#### The underlying implementation of unordered_map
The underlying implementation of unordered_map is based on the data structure of a hash table. When inserting an element, the hash value is first calculated based on the key, and then the value is stored at the corresponding position in the hash table. When looking up an element, the hash value is also calculated based on the key, and then the element at the corresponding position in the hash table is searched for. Due to the excellent design of the hash function, elements can be evenly distributed in the hash table, thus achieving fast lookup operations.
#### Characteristics of unordered_map
Unordered_map has the following characteristics:
- The time complexity of lookup, insertion, and deletion operations is O(1), which is very efficient.
- The storage of elements is unordered, and they will not be arranged in order of key size.
- Unordered_map uses a hash table to store data, which can provide high performance for large datasets.
- Compared with map, the iteration order of unordered_map is uncertain because the storage of elements is unordered.
# Basics of Concurrent Programming
#### What is Concurrent Programming
Concurrent programming refers to a programming paradigm where multiple computational tasks are performed simultaneously. In computer systems, multiple tasks are executed simultaneously through time-slicing rotation, as CPUs cannot truly process multiple tasks at the same time. The purpose of concurrent programming is to improve system utilization and performance.
Concurrent programming can
0
0
相关推荐
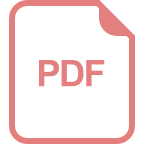
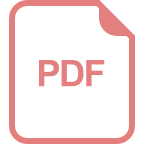
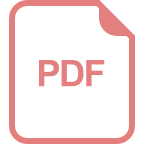





