The Clever Integration of unordered_map with Other STL Containers
发布时间: 2024-09-15 18:27:41 阅读量: 28 订阅数: 32 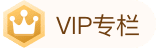
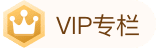
# 1. Introduction to STL Containers
In modern C++ programming, the Standard Template Library (STL) containers are indispensable tools. STL containers offer a wealth of data structure implementations, including arrays, linked lists, stacks, queues, and more. By leveraging STL containers, developers can enhance the efficiency and maintainability of their code, allowing them to concentrate on implementing business logic rather than getting bogged down by the intricacies of underlying data structures.
The design of STL containers takes into account performance, flexibility, and ease of use, making them a vital component of C++ programming. Familiarity with the characteristics and usage of STL containers can greatly assist developers in solving problems more effectively and improving the quality and scalability of their code. In this chapter, we will delve into the concepts, features, and applications of STL containers, providing readers with a comprehensive understanding.
# 2. Detailed Explanation of unordered_map
### Features of unordered_map
The unordered_map is one of the associative containers in the C++ Standard Template Library, implemented using a hash table, which facilitates fast lookup, insertion, and deletion operations. Unlike its counterpart, the map, elements in an unordered_map are stored in an unordered manner and are not sorted based on key values.
### Example Usage of unordered_map
Here is a simple example demonstrating the use of an unordered_map for inserting, searching, and removing elements:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> umap;
// Insert key-value pairs
umap["apple"] = 5;
umap["banana"] = 3;
umap["orange"] = 7;
// Search for an element
if (umap.find("apple") != umap.end()) {
std::cout << "apple is found. Value: " << umap["apple"] << std::endl;
}
// Remove an element
umap.erase("banana");
// Iterate over the unordered_map
for (auto& pair : umap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
### Principles of unordered_map Implementation
The internal workings of an unordered_map are based on a hash table, which uses a hash function to map keys to buckets. Within these buckets, data structures such as linked lists or red-black trees are employed to handle collisions. When the number of elements increases, and the load factor exceeds a threshold, the unordered_map undergoes a rehash operation, reallocating storage space to maintain efficient operations. Therefore, a good hash function and an appropriate load factor are crucial for the performance of an unordered_map.
Through the above examples and explanations, we can see the usage methods and characteristics of an unordered_map, as well as its underlying implementation principles. In actual development, selecting the right container is essential for enhancing program performance and efficiency.
# 3. Applications of STL Containers
In practical development, we often need to choose the appropriate STL container based on different requirements. Each STL container shines in various application scenarios, and we will discuss the use cases of array containers, queue containers, and stack containers separately.
### Advantages and Applications of Array Containers
Arrays are one of the most fundamental data structures, and in STL, they are encapsulated as `std::array`, which provides many convenient operation functions. Array containers are suitable for scenarios that require fast random access to elements since arrays are stored contiguously in memory, allowing for quicker access.
Usi
0
0
相关推荐








