unordered_map Size and Capacity Operations Analysis
发布时间: 2024-09-15 18:21:28 阅读量: 20 订阅数: 26 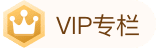
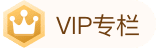
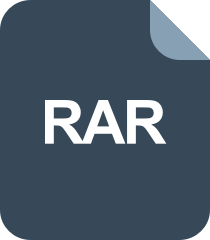
C++11 unordered_map与map(插入,遍历,Find)效率对比。
# 1.1 Understanding unordered_map
In the C++ STL, `unordered_map` is an associative container that utilizes a hash table as its underlying data structure. The hash table maps key-value pairs to buckets using a hash function, facilitating rapid lookups, insertions, and deletions. `unordered_map` is suitable for scenarios that require quick access to key-value pairs, such as storing unique keys with their corresponding values. It offers constant time complexity for search, insertion, and deletion operations, providing fast performance. Using `unordered_map` allows for the quick定位 of elements without the need for traversing the entire container in a specific order. This is particularly important for large-scale data storage and retrieval, ensuring that programs maintain high efficiency when dealing with vast amounts of data.
One of the characteristics of `unordered_map` is that its elements are stored in an unordered manner, and the order of the key-value pairs depends on the outcomes of the hash function. Therefore, if the order of elements is a requirement, other ordered containers should be chosen for data storage.
# 2. Construction and Initialization of unordered_map
The construction and initialization of `unordered_map` is the first step in using this data structure, and an appropriate construction method can enhance the efficiency and readability of the program.
#### 2.1 Creating an unordered_map Object
The creation of an unordered associative container can be achieved using either the default constructor or parameterized constructors.
##### 2.1.1 Default Constructor
The default constructor will create an empty `unordered_map` object, as shown in the example code below:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap;
return 0;
}
```
##### 2.1.2 Parameterized Constructor
The parameterized constructor accepts an initial size and optional hash function and key equality function, for instance:
```cpp
std::unordered_map<int, std::string> myMap(10); // Initial size is 10
```
#### 2.2 Initializing an unordered_map
An `unordered_map` can be initialized using an initializer list `{}`, the `insert` method, or the `emplace` method.
##### 2.2.1 Using an Initializer List
An `unordered_map` can be initialized with key-value pairs using an initializer list, as shown in the example code below:
```cpp
std::unordered_map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
```
##### 2.2.2 Usage of the insert Method
The `insert` method can be used to add elements to an `unordered_map`, as demonstrated in the example code below:
```cpp
myMap.insert({4, "Four"});
```
##### 2.2.3 Usage of the emplace Method
The `emplace` method can construct key-value pairs directly from its parameters and insert them into the `unordered_map`, as shown in the example code below:
```cpp
myMap.emplace(5, "Five");
```
When constructing and initializing an `unordered_map`, choosing the appropriate construction method can help improve program efficiency and readability, laying a foundation for subsequent operations.
# 3. Element Access in unordered_map
`unordered_map` is an associative container in the C++ STL that offers fast lookup, insertion, and deletion operations. When using an `unordered_map`, it's essential to understand how to access and modify its elements. This chapter will introduce the operations for accessing and modifying elements in an `unordered_map`.
#### 3.1 Accessing Elements
In an `unordered_map`, elements can be accessed by their keys. There are two common methods for acces
0
0
相关推荐
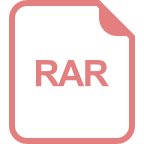
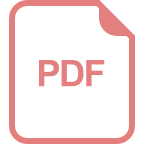
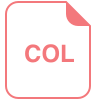
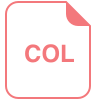



