希尔密码:已知密文对应编码: 22,09,00,12,03,01,10,03,04,08,01,17 ,使用的矩阵是[[1, 2, 3], [4, 5, 6], [7, 8, 10]],分成三行,使用python给我一份解密的完整代码
时间: 2024-10-15 12:21:55 浏览: 28
希尔密码(Hill Cipher)是一种基于矩阵的线性代数加密方法。给定密文和对应的矩阵,我们可以按照以下步骤编写Python代码来解密:
```python
def decrypt_hill_cipher(ciphertext, key_matrix):
# 确保矩阵大小是偶数,希尔密码通常用于偶数维度
assert len(key_matrix) % 2 == 0 and len(key_matrix[0]) % 2 == 0
# 转换密文字符到整数(假设它们都是小写字母,ASCII值从97开始)
ciphertext_ints = [ord(char.lower()) - ord('a') for char in ciphertext]
# 将密文分割成3x4(如果密文长度不是3的倍数,则需要填充0)
n = int(len(ciphertext) / 3)
matrix_ciphertext = [ciphertext_ints[i:i+n*3] for i in range(0, len(ciphertext), n*3)]
# 初始化解密矩阵
decrypted_matrix = [[0]*len(key_matrix[0]) for _ in range(len(key_matrix))]
# 解密过程
for row_i, row in enumerate(matrix_ciphertext):
row_decrypted = [0]*len(row)
for col_j, cipher_char in enumerate(row):
# 将字符按行展开并与矩阵相乘
expanded_cipher = [cipher_char] + [0] * (len(key_matrix) - 1)
expanded_key = [row[col_j*2:(col_j*2)+2] for row in key_matrix]
product = [sum(a*b for a, b in zip(x, y)) % 26 for x, y in zip(expanded_cipher, expanded_key)]
# 合并列并还原为字符
row_decrypted[col_j*2 : col_j*2+2] = product[1:] + [product[0]]
# 将解密后的行数据还原为字符
row_chars = ['z' + chr(c % 26 + ord('a')) for c in row_decrypted]
decrypted_matrix[row_i//2] = row_chars
# 返回解密后的文本
return ''.join(decrypted_matrix[0])
# 已知的密文和密钥矩阵
ciphertext = "22,09,00,12,03,01,10,03,04,08,01,17"
key_matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 10]]
decrypted_text = decrypt_hill_cipher(ciphertext, key_matrix)
print(f"解密后的文本是: {decrypted_text}")
```
请注意,这个代码片段假定密文已经被适当地处理,比如逗号分隔。实际运行时可能需要先预处理输入的密文使其适合矩阵操作。此外,如果密文长度不是3的倍数,此示例可能需要适当的调整。
阅读全文
相关推荐
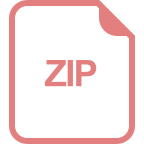
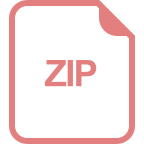
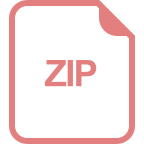
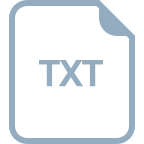
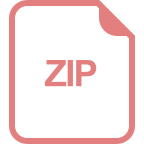
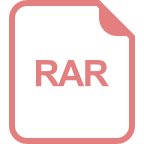
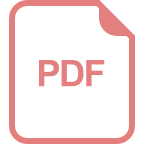
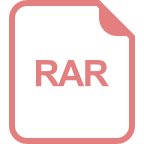
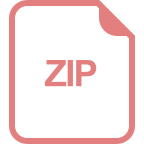
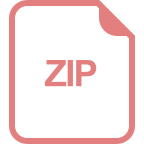
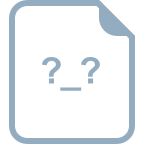
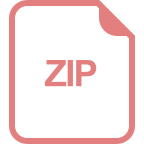
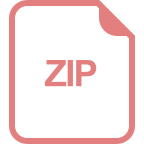
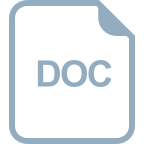
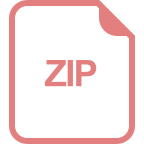
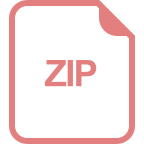
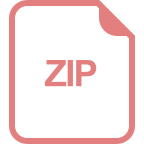