javaee完成多条件(根据姓名,年龄和性别)查询员工的功能,要求使用动态sql
时间: 2024-09-09 22:10:56 浏览: 62
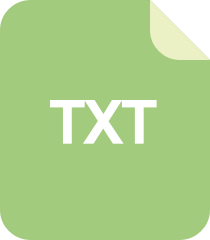
JavaEE源码企业员工信息管理源码数据库sql文档
在JavaEE中实现多条件动态查询员工的功能,通常会涉及到几个技术组件,比如MyBatis框架以及其动态SQL功能。下面是一个基于MyBatis框架的简单示例,展示如何构建这样一个查询功能:
首先,你需要定义一个EmployeeMapper接口,其中包含一个根据姓名、年龄和性别查询员工的方法:
```java
public interface EmployeeMapper {
List<Employee> selectEmployeeByCondition(@Param("name") String name,
@Param("age") Integer age,
@Param("gender") String gender);
}
```
然后,在EmployeeMapper.xml中使用动态SQL来构建查询语句。MyBatis的动态SQL元素包括`<if>`, `<choose>`, `<when>`, `<otherwise>`, `<where>`, `<set>`等,可以实现复杂的条件逻辑。
```xml
<mapper namespace="com.example.mapper.EmployeeMapper">
<select id="selectEmployeeByCondition" resultType="com.example.entity.Employee">
SELECT * FROM employees
<where>
<if test="name != null and name != ''">
AND name LIKE CONCAT('%', #{name}, '%')
</if>
<if test="age != null">
AND age = #{age}
</if>
<if test="gender != null and gender != ''">
AND gender = #{gender}
</if>
</where>
</select>
</mapper>
```
在这个SQL查询中,`<where>`标签会自动添加一个WHERE关键字,并且只有当`<if>`条件满足时才会添加相应的SQL条件,避免了AND关键字的多余出现。使用CONCAT函数是为了实现对姓名的模糊匹配,而`<if>`元素用于检查参数是否为null或空,确保只有提供了有效参数时才会在查询中使用它们。
最后,你需要在服务层或者业务逻辑层调用这个Mapper方法,传入相应的条件参数。
```java
@Service
public class EmployeeService {
@Autowired
private EmployeeMapper employeeMapper;
public List<Employee> getEmployees(String name, Integer age, String gender) {
return employeeMapper.selectEmployeeByCondition(name, age, gender);
}
}
```
阅读全文
相关推荐
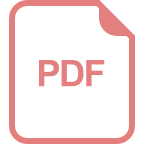
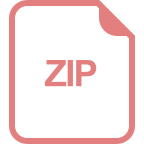
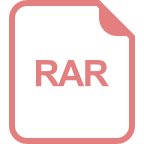
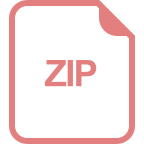
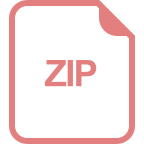
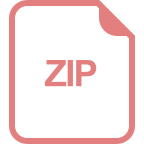
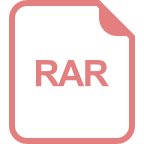
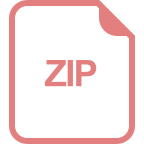
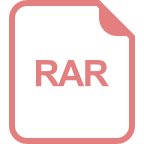
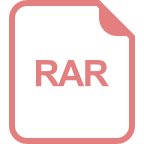
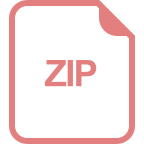
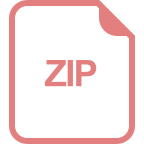
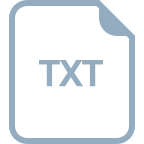
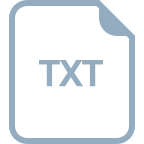
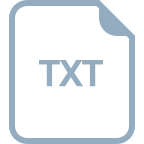
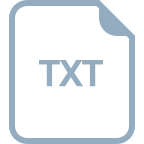
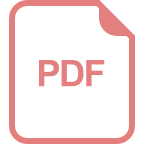