Modify your send.py program to send all the files in the “samples” directory for classification and print out the results
时间: 2024-04-12 12:28:47 浏览: 137
Sure! Here's the modified `send.py` program that sends all the files in the "samples" directory for classification and prints out the results:
```python
import paho.mqtt.client as mqtt
import numpy as np
import json
import os
# Insert model and classification code here
# Connection function
def on_connect(client, userdata, flags, rc):
if rc == 0:
print("Successfully connected to broker.")
else:
print("Connection failed with code: %d." % rc)
# Message handling
def on_message(client, userdata, msg):
recv_dict = json.loads(msg.payload)
filename = recv_dict["filename"]
img_data = np.array(recv_dict["data"])
result = classify_flower(filename, img_data)
print("Classification result for", filename, ":", result)
# Setup MQTT client
def setup(hostname):
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.connect(hostname)
client.loop_start()
return client
def main():
client = setup("172.17.0.3")
# Send all files in the "samples" directory for classification
samples_dir = "samples"
for filename in os.listdir(samples_dir):
file_path = os.path.join(samples_dir, filename)
if os.path.isfile(file_path):
with open(file_path, "rb") as f:
img_data = f.read()
payload = {
"filename": filename,
"data": img_data.tolist()
}
client.publish("Group_04/IMAGE/classify", json.dumps(payload))
while True:
pass
if __name__ == '__main__':
main()
```
In this modified version of `send.py`, we iterate over all the files in the "samples" directory using `os.listdir()` and `os.path.join()` functions
阅读全文
相关推荐




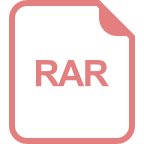

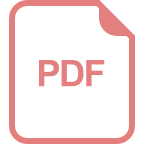




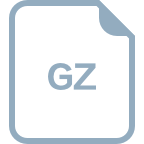

