在linux中用python3写一个用fio测试固态硬盘的python脚本: (1)对待测试盘进行擦除 (2)使用fio进行128K顺序读写测试,并记录测试结果为test_FIO_128K.log (3)用[line for line in fio_reasults.split("\n") if "BW=" in line]类似的语法提取测试结果中的BW、IOPS、lat (usec)到excel中 (4)使用logging模块将步骤(1)(2)(3)记录在测试log中,并同步打印出调试信息
时间: 2024-04-15 11:30:20 浏览: 414
你可以使用以下代码来实现这个需求:
```python
import os
import subprocess
import logging
import re
import xlwt
def erase_drive(device):
logging.info("Erasing drive: %s", device)
subprocess.run(["blkdiscard", device])
def run_fio_test(device, log_file):
logging.info("Running FIO test on device: %s", device)
subprocess.run(["fio", "--name=test", "--filename=" + device, "--rw=write", "--bs=128k", "--size=1G", "--output-format=json", "--output=" + log_file])
def extract_results(log_file, excel_file):
logging.info("Extracting results from log file: %s", log_file)
with open(log_file, "r") as f:
fio_results = f.read()
bw_pattern = r"BW=(\d+\.\d+ \w+/s)"
iops_pattern = r"IOPS=(\d+)"
lat_pattern = r"lat.*?avg=(\d+)"
bw_match = re.search(bw_pattern, fio_results)
iops_match = re.search(iops_pattern, fio_results)
lat_match = re.search(lat_pattern, fio_results)
if bw_match and iops_match and lat_match:
bw = bw_match.group(1)
iops = iops_match.group(1)
latency = lat_match.group(1)
logging.info("BW: %s, IOPS: %s, Latency: %s", bw, iops, latency)
workbook = xlwt.Workbook()
sheet = workbook.add_sheet("Results")
sheet.write(0, 0, "BW")
sheet.write(0, 1, "IOPS")
sheet.write(0, 2, "Latency (usec)")
sheet.write(1, 0, bw)
sheet.write(1, 1, iops)
sheet.write(1, 2, latency)
workbook.save(excel_file)
else:
logging.error("Failed to extract results from log file")
def setup_logging():
logging.basicConfig(level=logging.DEBUG,
format="%(asctime)s %(levelname)s %(message)s",
handlers=[
logging.FileHandler("test.log"),
logging.StreamHandler()
])
def main():
device = "/dev/sdb" # 替换为待测试的硬盘设备路径
log_file = "test_FIO_128K.log"
excel_file = "results.xls"
setup_logging()
erase_drive(device)
run_fio_test(device, log_file)
extract_results(log_file, excel_file)
if __name__ == "__main__":
main()
```
请确保你已经安装了 `fio` 和 `xlwt` 模块。运行这个脚本时,它会执行以下操作:
1. 擦除指定的硬盘设备。
2. 在指定的硬盘设备上运行 FIO 测试,并将结果保存到日志文件中。
3. 从日志文件中提取带宽、IOPS 和延迟(usec)的测试结果,并将其保存到 Excel 文件中。
4. 使用 `logging` 模块将步骤(1)、(2)和(3)记录在测试日志中,并同时打印调试信息。
你可以根据需要修改硬盘设备路径和文件名。
阅读全文
相关推荐
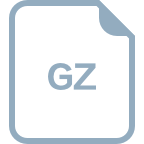















