c语言译密码字母变成前4个
时间: 2024-10-24 07:09:52 浏览: 26
在C语言中,将字母密码转换成前四个字母可以创建一个简单的替换算法,通常用于凯撒密码(Caesar Cipher)的一种简化版本。这个过程会遍历输入字符串,取出每个字符,然后取其ASCII码值,对特定偏移量进行处理,然后再转换回字符。以下是基本的实现步骤:
```c
#include <stdio.h>
#include <string.h>
char* translate_to_four_chars(char* password) {
int len = strlen(password);
char result[len];
for (int i = 0; i < len; i++) {
// 检查是否是英文字母
if ('A' <= password[i] && password[i] <= 'Z') {
result[i] = ((password[i] - 'A' + 4) % 26) + 'A';
} else if ('a' <= password[i] && password[i] <= 'z') {
result[i] = ((password[i] - 'a' + 4) % 26) + 'a';
} else {
// 非字母字符不变
result[i] = password[i];
}
}
result[len] = '\0'; // 添加终止符
return result;
}
int main() {
char input_password[] = "AbCdeFgH";
char* transformed_password = translate_to_four_chars(input_password);
printf("Translated password: %s\n", transformed_password);
// 清理内存
free(transformed_password);
return 0;
}
```
在这个例子中,`translate_to_four_chars`函数会将输入字符串的每一个字母向前移动4位,如果超过'Z'或'z',则返回循环到开始位置。
阅读全文
相关推荐
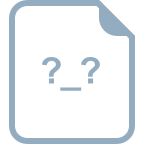
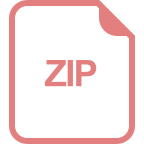
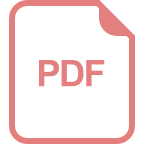

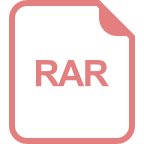














