winform读取OpenFileDialog
时间: 2023-12-17 18:04:12 浏览: 84
在Winform中读取OpenFileDialog的过程如下:
1. 首先,在Winform的按钮点击事件中创建一个OpenFileDialog实例:OpenFileDialog fileDialog = new OpenFileDialog();
2. 然后,设置OpenFileDialog的相关属性,比如是否允许选择多个文件、对话框标题和文件筛选器:fileDialog.Multiselect = true; fileDialog.Title = "请选择文件"; fileDialog.Filter = "所有文件(*xls*)|*.xls*";
3. 接下来,判断用户是否选择了文件并点击了确定按钮:if (fileDialog.ShowDialog() == DialogResult.OK)
4. 如果用户选择了文件并点击了确定按钮,可以通过fileDialog.FileName属性获取文件的完整路径:localFilePath = fileDialog.FileName;
5. 最后,你可以使用所选择的文件路径进行后续的操作,比如显示文件路径或处理文件。
相关问题
winform openfiledialog excel
好的,你想要在 WinForm 中使用 OpenFileDialog 打开 Excel 文件,对吧?你可以按照以下步骤进行操作:
1. 首先,你需要在 WinForm 中添加 OpenFileDialog 控件。你可以在工具箱中找到 OpenFileDialog 控件,然后将它拖动到窗体中。
2. 接着,在你想要使用 OpenFileDialog 的按钮的 Click 事件中添加以下代码:
```
OpenFileDialog openFileDialog1 = new OpenFileDialog();
openFileDialog1.Filter = "Excel Files|*.xls;*.xlsx;*.xlsm";
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
string filePath = openFileDialog1.FileName;
// 进行Excel文件的读取操作
}
```
这段代码会打开一个 OpenFileDialog 窗口,允许用户选择 Excel 文件,并返回选择的文件路径。你可以在这个路径下进行 Excel 文件的读取操作。
3. 最后,你需要添加对 Microsoft.Office.Interop.Excel 的引用,以便在代码中访问 Excel 文件。你可以在 Visual Studio 中右键点击项目,选择“添加引用”,然后在“COM”选项卡中找到并勾选相应的 Microsoft Excel 库。
希望这些步骤能够对你有所帮助!
winform读取csv
要在 WinForm 中读取 CSV 文件,可以使用以下步骤:
1. 引入 System.IO 命名空间,使用 StreamReader 类读取文件。
2. 使用 OpenFileDialog 控件选择要读取的 CSV 文件。
3. 读取文件时,使用逗号作为分隔符,将每行数据分割成一个字符串数组。
4. 将每行数据转换为需要的数据类型,可以使用 Convert 类或者自定义方法。
5. 将数据绑定到 WinForm 控件上,例如 DataGridView。
下面是一个示例代码:
```csharp
using System.IO;
// ...
private void btnOpenFile_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "CSV files (*.csv)|*.csv";
openFileDialog.RestoreDirectory = true;
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
try
{
using (StreamReader reader = new StreamReader(openFileDialog.FileName))
{
string line;
while ((line = reader.ReadLine()) != null)
{
string[] fields = line.Split(',');
// TODO: 将每行数据转换为需要的数据类型
// TODO: 将数据绑定到 WinForm 控件上
}
}
}
catch (Exception ex)
{
MessageBox.Show("Error reading CSV file: " + ex.Message);
}
}
}
```
在上面的代码中,btnOpenFile_Click 是一个按钮的 Click 事件处理程序,用于打开选择 CSV 文件的对话框。可以根据需要修改代码,将数据绑定到 DataGridView 或其他 WinForm 控件上。
阅读全文
相关推荐
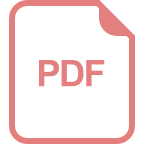
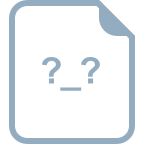
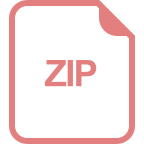
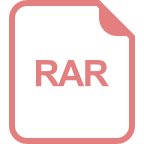
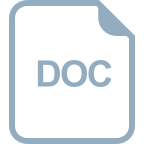










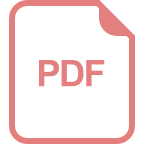
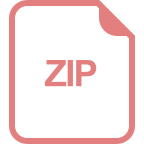