rust怎么实现Vec<u8> to [u8;32]
时间: 2024-09-21 13:06:18 浏览: 72
在Rust中,`Vec<u8>` 是一个动态大小的字节切片,而 `[u8; 32]` 则是一个固定大小的静态数组。如果你有一个长度已知并且小于32的`Vec<u8>`,可以将其转换为固定的`[u8; 32]`,但是需要注意长度的匹配。如果`Vec`的实际长度大于32,则无法直接转换,因为静态数组的大小是固定的。
这里提供一种简单的转换方法,前提是`Vec`的长度小于或等于32:
```rust
fn vec_to_fixed_array(vec: Vec<u8>) -> &[u8; 32] {
if vec.is_empty() || vec.len() <= 32 {
&vec[..32]
} else {
panic!("Cannot convert vector with length greater than 32");
}
}
fn main() {
let some_data: Vec<u8> = [1, 2, 3, 4, 5]; // 假设这是一个小于32的字节切片
let fixed_array = vec_to_fixed_array(some_data);
println!("{:?}", fixed_array); // 输出字节数组
}
```
上面的`vec_to_fixed_array`函数首先检查`Vec`是否为空或长度不大于32,如果是则安全地创建了一个大小为32的切片。如果超过这个限制,会触发一个panic,因为尝试超出范围访问数组是不允许的。
相关问题
用rust实现一个http的parse_request方法
为了实现一个 HTTP 请求解析的方法,你可以使用 Rust 的标准库中的 `http` 包。
首先,你需要将请求字符串解析为 `http::Request<Vec<u8>>` 类型的实例。 你可以使用 `http::Request::try_from` 方法将请求字符串解析为一个 `http::Request` 实例。
例如:
```rust
use http::{Request, Method};
fn parse_request(request_str: &str) -> Result<Request<Vec<u8>>, http::Error> {
let request = Request::try_from(request_str)?;
Ok(request)
}
```
你也可以使用 `http::request::Builder` 来构建一个 `http::Request` 实例。例如:
```rust
use http::{Request, Method};
fn parse_request(request_str: &str) -> Result<Request<Vec<u8>>, http::Error> {
let request = Request::builder()
.method(Method::GET)
.uri("/")
.body(Vec::new())
.unwrap();
Ok(request)
}
```
在上面的代码中,我们使用了 `http::Request::builder` 方法来创建一个新的请求构建器,然后使用 `method`、`uri` 和 `body` 方法来设置请求的方法、URL 和正文。最后,我们使用 `unwrap` 方法来解析构建器为一个 `http::Request` 实例。
rust实现Brainfuck解释器的代码
我可以回答这个问题。以下是一个Rust实现的Brainfuck解释器的代码示例:
```rust
use std::io::{self, Read};
const MEMORY_SIZE: usize = 30000;
struct Interpreter {
memory: [u8; MEMORY_SIZE],
pointer: usize,
code: Vec<char>,
code_pointer: usize,
}
impl Interpreter {
fn new(code: Vec<char>) -> Interpreter {
Interpreter {
memory: [; MEMORY_SIZE],
pointer: ,
code,
code_pointer: ,
}
}
fn run(&mut self) {
while self.code_pointer < self.code.len() {
match self.code[self.code_pointer] {
'>' => self.pointer += 1,
'<' => self.pointer -= 1,
'+' => self.memory[self.pointer] += 1,
'-' => self.memory[self.pointer] -= 1,
'.' => print!("{}", self.memory[self.pointer] as char),
',' => {
let mut buffer = [];
io::stdin().read_exact(&mut buffer).unwrap();
self.memory[self.pointer] = buffer[];
}
'[' => {
if self.memory[self.pointer] == {
let mut count = 1;
while count > {
self.code_pointer += 1;
match self.code[self.code_pointer] {
'[' => count += 1,
']' => count -= 1,
_ => (),
}
}
}
}
']' => {
if self.memory[self.pointer] != {
let mut count = 1;
while count > {
self.code_pointer -= 1;
match self.code[self.code_pointer] {
'[' => count -= 1,
']' => count += 1,
_ => (),
}
}
}
}
_ => (),
}
self.code_pointer += 1;
}
}
}
fn main() {
let code = String::from("++++++++[>++++[>++>+++>+++>+<<<<-]>+>+>->>+[<]<-]>>.>---.+++++++..+++.>>.<-.<.+++.------.--------.>>+.>++.").chars().collect();
let mut interpreter = Interpreter::new(code);
interpreter.run();
}
```
希望这个代码示例能够帮助你!
阅读全文
相关推荐
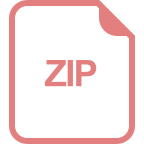
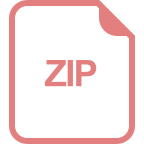
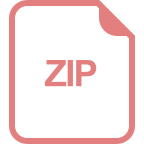













