Write a program that checks if a specific element exists in a tuple using a for loop.
时间: 2024-10-26 18:16:11 浏览: 16
To check if an element exists in a tuple using a `for` loop in Rust, you don't need to use a separate data structure like lists (`Option` or `Vec`) since tuples are immutable by default. Here's how you can do it:
```rust
// Define a tuple with some elements
let tuple: (u8, String, i32) = (42, "hello".to_string(), 1337);
fn contains_element(element: &u8, tup: &(_, _, _)) -> bool {
// Iterate over the tuple using a pattern matching for loop
for (e, _, _) in tup {
// Compare the current element with the target element
if *e == element {
return true; // Found the element, exit the loop and return true
}
}
false // Element not found after the loop, return false
}
// Check if an element exists in the tuple
if let Some(index) = Some(0) { // Assuming you have the index of the element to search
let element_to_find = 42;
if contains_element(&element_to_find, &tuple) {
println!("The element {} exists in the tuple.", element_to_find);
} else {
println!("The element {} does not exist in the tuple.", element_to_find);
}
}
```
In this example, the `contains_element` function takes a reference to the target element and a tuple as parameters. It iterates through the tuple using a pattern-matched `for` loop and checks if each element matches the target. If it finds a match, it returns `true`; otherwise, it returns `false`.
Note that the code assumes you already know the index of the element you want to search within the tuple. If you're dynamically searching for an element without an index, you might need to use a different approach.
阅读全文
相关推荐
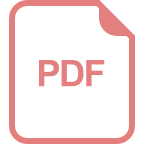
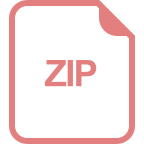
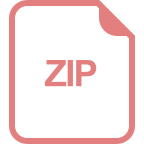















