how to split a docx file with images and tables by title using docx4j
时间: 2024-04-08 09:29:26 浏览: 130
To split a DOCX file with images and tables into multiple DOCX files by title using docx4j, you can follow these steps:
1. Load the original DOCX file using the docx4j library:
```java
WordprocessingMLPackage wordMLPackage = WordprocessingMLPackage.load(new File("path/to/original.docx"));
```
2. Iterate through the paragraphs in the document and find the paragraphs that contain the titles (e.g., using specific styles or formatting). You can use the `getMainDocumentPart().getContent()` method to access the paragraphs:
```java
List<Object> paragraphs = wordMLPackage.getMainDocumentPart().getContent();
for (Object obj : paragraphs) {
if (obj instanceof P) {
P paragraph = (P) obj;
// Check if the paragraph is a title (based on styles or formatting)
// Split the document based on your conditions
}
}
```
3. Once you identify the paragraphs containing the titles, create a new WordprocessingMLPackage for each section and copy the content into these new packages:
```java
WordprocessingMLPackage newPackage = WordprocessingMLPackage.createPackage();
newPackage.getMainDocumentPart().getContent().add(paragraph);
```
4. If the title paragraphs are followed by images or tables, you can iterate through the paragraph's content and check for images or tables using `instanceof` checks. Extract and copy the images to the new package's media folder, and copy the tables to the new package's main document part:
```java
for (Object obj : paragraph.getContent()) {
if (obj instanceof Drawing) {
Drawing drawing = (Drawing) obj;
List<Object> imageList = drawing.getAnchorOrInline();
for (Object image : imageList) {
if (image instanceof Inline) {
Inline inline = (Inline) image;
// Copy the image to the new package's media folder
}
}
} else if (obj instanceof Tbl) {
Tbl table = (Tbl) obj;
newPackage.getMainDocumentPart().getContent().add(table);
}
}
```
5. Save each new package as a separate DOCX file using the desired title:
```java
newPackage.save(new File("path/to/" + title + ".docx"));
```
Remember to handle any exceptions that may occur during this process. This is a general outline, and you may need to adapt the code based on your specific requirements and conditions for splitting the document.
阅读全文
相关推荐
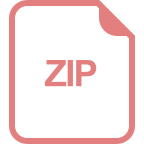
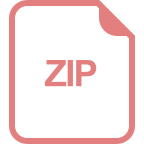
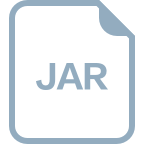



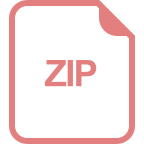
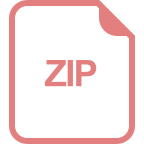
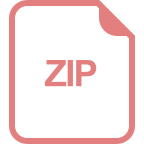
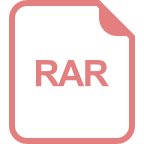
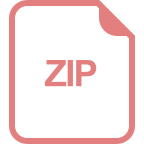
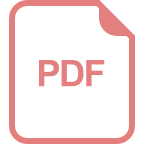
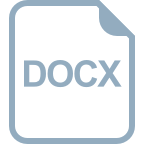
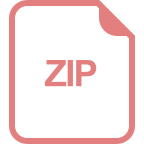