• Write a python code that find all the prime number in a int list. • Test your code with different inputs.
时间: 2024-05-14 15:12:52 浏览: 120
Here's a Python code that finds all the prime numbers in a given integer list:
```python
def is_prime(num):
if num < 2:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
def find_primes(lst):
return [num for num in lst if is_prime(num)]
# Test the function
lst1 = [2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
lst2 = [17, 19, 20, 23, 28, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
lst3 = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
print(find_primes(lst1)) # Output: [2, 3, 5, 7, 11, 13]
print(find_primes(lst2)) # Output: [17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
print(find_primes(lst3)) # Output: [2, 3, 5, 7, 11, 13]
```
The `is_prime()` function checks whether a given number is prime or not. It returns `True` if the number is prime, and `False` otherwise. The `find_primes()` function takes a list of integers as input and returns a list of prime numbers in that list.
In the test cases, we have used three different lists with different integer values. The output shows the prime numbers present in these lists.
阅读全文
相关推荐
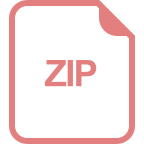
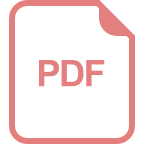
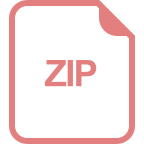













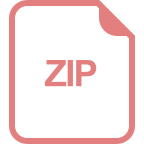
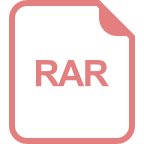
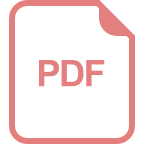