use c++ to write a program that prompts the user to enter two integers and checks whether the first number is divisible by the second.
时间: 2024-09-13 16:14:29 浏览: 81
当然可以。以下是一个简单的C++程序,它会提示用户输入两个整数,并检查第一个数字是否能被第二个数字整除:
```cpp
#include <iostream>
using namespace std;
int main() {
int num1, num2;
// 提示用户输入两个整数
cout << "请输入第一个整数:";
cin >> num1;
cout << "请输入第二个整数:";
cin >> num2;
// 检查第一个数字是否能被第二个数字整除
if (num2 != 0) { // 避免除数为零的错误
if (num1 % num2 == 0) {
cout << "第一个数 (" << num1 << ") 可以被第二个数 (" << num2 << ") 整除。" << endl;
} else {
cout << "第一个数 (" << num1 << ") 不能被第二个数 (" << num2 << ") 整除。" << endl;
}
} else {
cout << "第二个数不能为零,因为除数不能为零。" << endl;
}
return 0;
}
```
在这个程序中,我们首先获取用户的输入,然后通过`%`运算符(取余运算符)来判断第一个数是否能被第二个数整除。如果余数为0,则表示第一个数可以被第二个数整除。
相关问题
Write a program that prompts the user to enter an integer and reports whether the integer is a palindrome. A number is a palindrome if its reversal is the same as itself. Here are two sample runs: Enter an integer: 121 121 is a palindrome. Enter an integer:123 123 is not a palindrome.
Sure, here's a Python program that does what you're asking for:
```python
# Prompt the user to enter an integer
num = input("Enter an integer: ")
# Check if the integer is a palindrome
if num == num[::-1]:
print(f"{num} is a palindrome.")
else:
print(f"{num} is not a palindrome.")
```
Here's an explanation of how the program works:
1. We prompt the user to enter an integer using the `input()` function, and store the result in the variable `num`.
2. We check if `num` is equal to its reversal, which we can obtain by using the slicing operator `[::-1]` to reverse the string. If `num` is equal to its reversal, we print a message saying that it's a palindrome. Otherwise, we print a message saying that it's not a palindrome.
Note that we're treating the input as a string, since we want to be able to reverse it easily using string slicing. If we wanted to treat it as an integer instead, we would need to convert it using `int(num)` before reversing it.
Write the methods with the following headers // Return the reversal of an integer, i.e., reverse(456) returns 654 int reverse(int number) // Return true if number is a palindrome int isPalindrome(int number) Use the reverse method to implement isPalindrome. A number is a palindrome if its reversal is the same as itself. Write a test program that prompts the user to enter an integer and reports whether the integer is a palindrome.
Sure! Here are the methods you requested:
```java
public static int reverse(int number) {
int reversedNumber = 0;
while (number != 0) {
reversedNumber = reversedNumber * 10 + number % 10;
number /= 10;
}
return reversedNumber;
}
public static boolean isPalindrome(int number) {
return number == reverse(number);
}
```
And here's a program that uses these methods to check if a user-entered integer is a palindrome:
```java
import java.util.Scanner;
public class PalindromeChecker {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer: ");
int number = input.nextInt();
if (isPalindrome(number)) {
System.out.println(number + " is a palindrome");
} else {
System.out.println(number + " is not a palindrome");
}
}
}
```
Hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
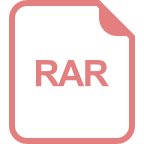
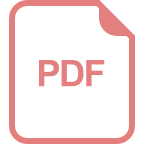
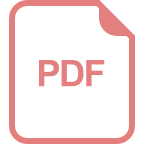











