(Algebra: perfect square) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number of times in the array list. Thus, n is 10.)
时间: 2023-07-16 11:16:00 浏览: 110
Here's a Python program that implements the algorithm described in the problem statement:
```
import math
def smallest_perfect_square_factor(m):
factors = []
# Find all smallest factors of m
while m % 2 == 0:
factors.append(2)
m = m // 2
for i in range(3, int(math.sqrt(m))+1, 2):
while m % i == 0:
factors.append(i)
m = m // i
if m > 2:
factors.append(m)
# Compute n as the product of factors that appear an odd number of times
odd_factors = [x for x in factors if factors.count(x) % 2 == 1]
n = 1
for f in odd_factors:
n *= f
return n
m = int(input("Enter an integer m: "))
n = smallest_perfect_square_factor(m)
print(f"The smallest integer n such that m * n is a perfect square is {n}.")
```
The program first computes all the smallest factors of `m` and stores them in an array list called `factors`. Then, it identifies the factors that appear an odd number of times in the list, and multiplies them together to obtain `n`. Finally, the program prints out the value of `n`.
阅读全文
相关推荐
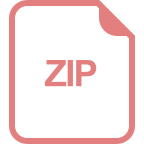
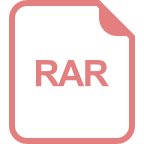
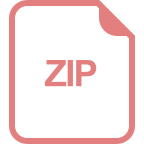


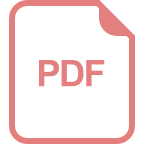
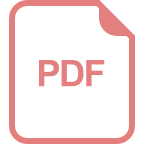
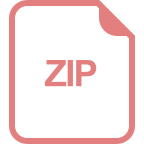






