Java(Algebra: perfect square) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number of times in the array list. Thus, n is 10.) Here are some sample runs: Enter an integer m: 1500 The smallest number n for m * n to be a perfect square is 15 m * n is 22500
时间: 2023-07-16 10:16:19 浏览: 178
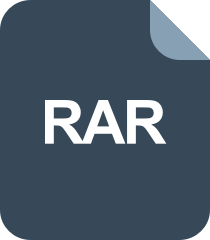
gauss_seidel.rar_To the Letter
Here's the Java program to solve the problem:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class PerfectSquare {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer m: ");
int m = input.nextInt();
// Find all smallest factors of m and store them in an array list
ArrayList<Integer> factors = new ArrayList<Integer>();
int factor = 2;
while (factor <= m) {
if (m % factor == 0) {
factors.add(factor);
m /= factor;
} else {
factor++;
}
}
// Find the product of the factors that appear an odd number of times
int n = 1;
for (int i = 0; i < factors.size(); i++) {
int count = 0;
for (int j = 0; j < factors.size(); j++) {
if (factors.get(i) == factors.get(j)) {
count++;
}
}
if (count % 2 != 0) {
n *= factors.get(i);
}
}
// Compute m * n and output the result
int product = m * n;
System.out.println("The smallest number n for m * n to be a perfect square is " + n);
System.out.println("m * n is " + product);
}
}
```
Explanation:
- We first prompt the user to enter an integer `m`.
- We then find all smallest factors of `m` and store them in an array list `factors`. We use a loop with a variable `factor` that starts from 2 and goes up to `m`. If `m` is divisible by `factor`, we add `factor` to the array list and divide `m` by `factor`. Otherwise, we increment `factor`.
- After we have all the factors in the array list, we need to find the product of the factors that appear an odd number of times. We use a nested loop to count the occurrences of each factor in the array list. If the count is odd, we multiply the factor to `n`.
- Finally, we compute `m * n` and output the result.
阅读全文
相关推荐
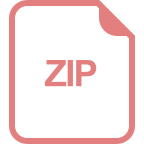
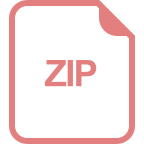















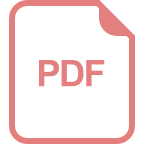