Java (Algebra: perfect square) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number of times in the array list. Thus, n is 10.)
时间: 2023-07-16 15:16:02 浏览: 47
Here is the Java code to implement the above program:
```
import java.util.ArrayList;
import java.util.Scanner;
public class PerfectSquare {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer m: ");
int m = input.nextInt();
ArrayList<Integer> factors = new ArrayList<>();
int factor = 2;
while (m > 1) {
if (m % factor == 0) {
factors.add(factor);
m /= factor;
} else {
factor++;
}
}
int n = 1;
for (int i = 0; i < factors.size(); i++) {
int count = 0;
for (int j = 0; j < factors.size(); j++) {
if (factors.get(i) == factors.get(j)) {
count++;
}
}
if (count % 2 != 0) {
n *= factors.get(i);
}
}
System.out.println("The smallest integer n such that m * n is a perfect square is " + n);
}
}
```
Explanation:
- We first prompt the user to enter an integer m using the Scanner class.
- We then create an ArrayList called factors to store all the smallest factors of m.
- We use a while loop to find all the smallest factors of m and add them to the ArrayList.
- We then use two nested for loops to find the factors that appear an odd number of times in the ArrayList and store their product in the variable n.
- Finally, we print out the value of n as the smallest integer such that m * n is a perfect square.
相关推荐
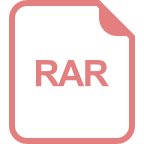
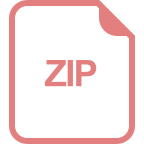














