(Sort ArrayList) Write the following method that sorts an ArrayList of numbers: public static void sort(ArrayList<Integer> list) Write a test program that prompts the user to enter five numbers, stores them in an array list, and displays them in increasing order.
时间: 2023-07-15 21:15:49 浏览: 115
Here's the implementation of the method and the test program:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
public class SortArrayList {
public static void sort(ArrayList<Integer> list) {
Collections.sort(list);
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
ArrayList<Integer> list = new ArrayList<>();
System.out.print("Enter 5 numbers: ");
for (int i = 0; i < 5; i++) {
list.add(input.nextInt());
}
sort(list);
System.out.println("Sorted numbers: " + list);
}
}
```
In the `sort` method, we use the `Collections.sort` method to sort the list in increasing order.
In the `main` method, we prompt the user to enter 5 numbers and store them in the `ArrayList` named `list`. Then we call the `sort` method to sort the list and display the sorted numbers.
阅读全文
相关推荐
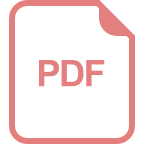





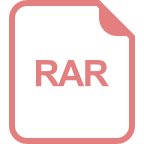
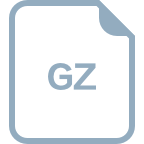









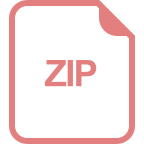