编写一个程序从键盘输入一个日期,要求计算该日期是这一年的第几天
时间: 2024-12-20 19:30:53 浏览: 6
编写这样的程序涉及到日期相关的计算,通常需要借助编程语言提供的日期库函数。这里提供一个简单的Python示例:
```python
import datetime
def calculate_day_of_year():
# 获取用户输入的日期字符串,格式可以是 'YYYY-MM-DD'
input_date_str = input("请输入日期(格式: YYYY-MM-DD): ")
# 将输入字符串转换为datetime对象
date_obj = datetime.datetime.strptime(input_date_str, '%Y-%m-%d')
# 使用date()函数获取纯日期部分,然后用today()函数获取今天的日期
today = datetime.date.today()
# 计算今年的第一天(1月1日)到用户输入日期之间的天数差
days_in_year = (today - date_obj.replace(year=today.year)) + datetime.timedelta(days=1)
return days_in_year.days
day_of_year = calculate_day_of_year()
print(f"{input_date_str}是{today.year}年的第{day_of_year}天")
#
相关问题
编写一个c语言程序从键盘输入一个日期,要求计算该日期是这一年的第几天
编写C语言程序来计算给定日期是一年中的第几天,通常涉及到闰年的判断。以下是简单的步骤和代码示例:
1. 定义一个函数,接受年份、月份和日期作为参数。
2. 判断是否是闰年。闰年规则:能被4整除的年份是闰年,但是能被100整除的不是,除非同时也能被400整除。
3. 计算每个月的天数,考虑二月特殊情况(平年28天,闰年29天)。
4. 根据给定的日期逐月累加天数。
下面是一个基础的代码示例:
```c
#include <stdio.h>
// Function to check if a year is leap
int is_leap(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// Calculate days in each month
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2:
return is_leap(year) ? 29 : 28;
default:
return -1; // Invalid month
}
}
// Main function
int main() {
int year, month, day;
printf("Enter the date (YYYY-MM-DD): ");
scanf("%d-%d-%d", &year, &month, &day);
// Ensure input is valid
if(month > 12 || day > days_in_month(month, year)) {
printf("Invalid date.\n");
return 1;
}
int total_days = 0;
for(int i = 1; i < month; ++i) {
total_days += days_in_month(i, year);
}
total_days += day;
printf("The given date is the %dth day of %d.\n", total_days, year);
return 0;
}
```
编写程序从键盘输入一个日期,要求计算输出该日期是这一年的第几天
### 回答1:
以下是Python代码实现:
```python
date_str = input("请输入日期(格式为YYYY-MM-DD):")
year, month, day = map(int, date_str.split("-"))
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29 # 闰年2月有29天
total_days = sum(days_in_month[:month-1]) + day
print(f"{year}年{month}月{day}日是这一年的第{total_days}天。")
```
代码解释:
1. 首先从键盘输入日期字符串,使用`split`方法将其拆分为年、月、日三个整数。
2. 定义一个列表`days_in_month`,存储每个月的天数。默认情况下2月有28天。
3. 判断输入的年份是否为闰年,如果是,则将`days_in_month`列表中2月的天数改为29。
4. 计算输入日期是这一年的第几天,即前面所有月份的天数之和加上当前月份的天数。
5. 使用`print`函数输出结果。
示例输出:
```
请输入日期(格式为YYYY-MM-DD):2022-03-15
2022年3月15日是这一年的第74天。
```
### 回答2:
本题的解决方案需要借助闰年和平年的概念,以及通过程序从键盘输入一个日期,并将其分离为年、月、日的数字。
首先,根据闰年和平年的规则,闰年指的是能够被4整除同时不能被100整除,或者是能够被400整除的年份;平年则为不符合这一条件的年份。
其次,要求将从键盘输入的日期拆分为年、月、日三个数字,可以使用字符串切片的方法将日期字符串划分为子字符串,然后通过类型转换将其转换为整型数值。例如,假设输入的日期格式为YYYY-MM-DD,则可以使用以下代码来分离日期:
```
date_str = input("请输入日期,格式为YYYY-MM-DD:")
year = int(date_str[:4])
month = int(date_str[5:7])
day = int(date_str[8:])
```
最后,根据每个月的天数以及闰平年的规则,可以编写程序计算出该日期是这一年的第几天。计算公式为:
```
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
days_per_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_per_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
total_days = sum(days_per_month[:month-1]) + day
print("该日期是这一年的第{}天。".format(total_days))
```
在以上代码中,通过判断年份是否为闰年来确定每个月的天数列表(days_per_month),然后使用列表切片的方式将该月之前的所有天数相加,并加上当月的天数,即为该日期是这一年的第几天。
以上是编写程序从键盘输入一个日期,计算输出该日期是这一年的第几天的方法介绍和实现代码。
### 回答3:
编写程序实现从键盘输入一个日期,可以按照以下步骤实现:
1. 首先,提示用户输入一个日期,要求日期格式为“年-月-日”,例如“2022-08-01”。
2. 接着,为了提取出日期中的年、月和日,可以使用字符串的切割(substring)功能,或者使用正则表达式的匹配(match)功能。具体实现方法取决于编程语言。
3. 提取出年、月和日之后,可以通过以下公式计算出该日期是这一年的第几天:
```
day_of_year = day + sum(days_in_month[1:month-1])
```
其中,`day`表示日期中的日,`month`表示日期中的月,`days_in_month`是一个列表,用于存储每个月份的天数。例如,对于平年,`days_in_month=[31,28,31,30,31,30,31,31,30,31,30,31]`。
4. 最后,输出计算得到的结果,即该日期是这一年的第几天。
需要注意的是,对于闰年,二月份的天数为29天。因此,需要根据闰年判断的规则,确定该年是否是闰年。通常,闰年的判断规则如下:
1. 能被4整除但不能被100整除的年份是闰年,如2004年就是闰年,1900年不是闰年。
2. 能被400整除的年份也是闰年,如2000年是闰年。
阅读全文
相关推荐
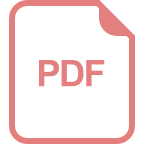
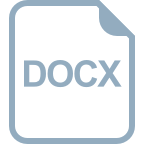
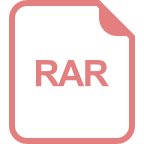













