yolov5-7.0调用摄像头的代码
时间: 2024-12-26 16:23:41 浏览: 6
### YOLOv5 7.0 调用摄像头 Python 示例代码
为了使用YOLOv5版本7.0调用摄像头并执行实时目标检测,可以采用Python脚本实现这一功能。下面提供了一个具体的例子来展示如何操作:
```python
import torch
from utils.general import non_max_suppression, scale_coords
from models.experimental import attempt_load
import cv2
import numpy as np
def letterbox(img, new_shape=(640, 640), color=(114, 114, 114)):
shape = img.shape[:2] # current shape [height, width]
r = min(new_shape[0] / shape[0], new_shape[1] / shape[1])
ratio = r, r # width, height ratios
new_unpad = int(round(shape[1] * r)), int(round(shape[0] * r))
dw, dh = new_shape[1] - new_unpad[0], new_shape[0] - new_unpad[1] # wh padding
dw /= 2 # divide padding into 2 sides
dh /= 2
if shape[::-1] != new_unpad: # resize
img = cv2.resize(img, new_unpad, interpolation=cv2.INTER_LINEAR)
top, bottom = int(round(dh - 0.1)), int(round(dh + 0.1))
left, right = int(round(dw - 0.1)), int(round(dw + 0.1))
img = cv2.copyMakeBorder(
img, top, bottom, left, right, cv2.BORDER_CONSTANT, value=color
) # add border
return img, ratio, (dw, dh)
device = 'cuda' if torch.cuda.is_available() else 'cpu'
model = attempt_load('yolov5s.pt', map_location=device) # 加载预训练模型[^1]
cap = cv2.VideoCapture(0) # 打开默认摄像头
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
img = letterbox(frame)[0]
img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, to 3x416x416
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).to(device)
img = img.float() # uint8 to fp16/32
img /= 255.0 # 归一化处理
if img.ndimension() == 3:
img = img.unsqueeze(0)
pred = model(img)[0]
pred = non_max_suppression(pred, conf_thres=0.25, iou_thres=0.45)
for i, det in enumerate(pred): # detections per image
gn = torch.tensor(frame.shape)[[1, 0, 1, 0]] # normalization gain whwh
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{conf:.2f}'
plot_one_box(xyxy, frame, label=label, color=[0, 255, 0]) # 绘制边界框
cv2.imshow("Frame", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
此段代码实现了通过摄像头捕获视频流,并利用加载好的YOLOv5模型对其进行逐帧的目标检测。对于每一帧图像,在完成必要的预处理之后将其送入模型预测;接着对输出的结果应用非极大值抑制(NMS),从而筛选出高质量的候选区域;最后将这些区域映射回原图尺寸,并在画面上标注出来。
阅读全文
相关推荐
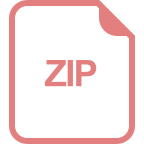
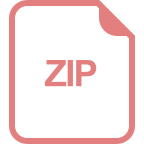
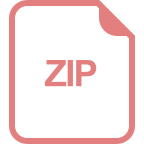
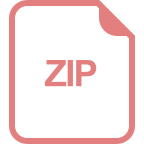
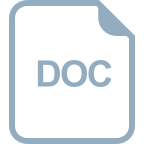












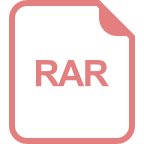