c++如何找出数据量不确定的数组中的最大值用max函数
时间: 2024-10-24 14:11:11 浏览: 24
在C++中,如果有一个动态大小或不确定大小的数组,并且你想找到其中的最大值,你可以先创建一个变量来存储当前已知的最大值,然后遍历数组,每次遇到更大的元素就更新这个变量。这不需要直接使用`std::max`函数,而是手动进行比较。
假设我们有一个`std::vector<int>`这样的动态数组:
```cpp
#include <iostream>
#include <vector>
int findMax(std::vector<int>& arr) {
if(arr.empty()) {
throw std::runtime_error("Array is empty");
}
int max_val = arr[0]; // 初始最大值设为第一个元素
for(size_t i = 1; i < arr.size(); ++i) {
if(arr[i] > max_val) {
max_val = arr[i];
}
}
return max_val;
}
int main() {
std::vector<int> numbers = {4, 8, 2, 16, 5};
try {
int max_number = findMax(numbers);
std::cout << "The maximum value in the array is: " << max_number << std::endl;
} catch(const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
在这个例子中,`findMax`函数接受一个`std::vector<int>`作为输入,通过迭代数组并逐个比较元素找到最大值。
阅读全文
相关推荐
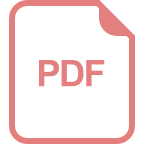
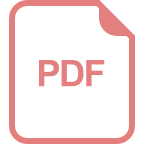
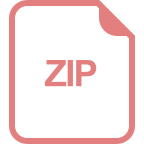
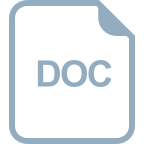
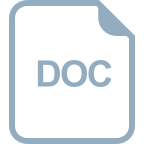
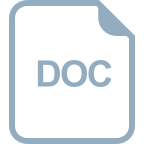
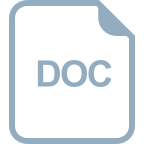
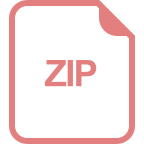
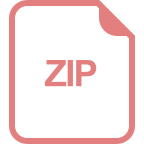
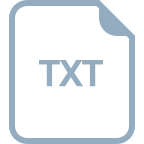
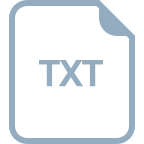
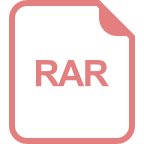
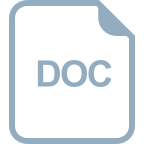
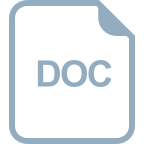
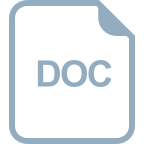
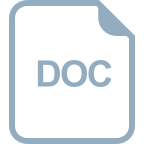
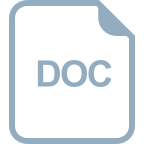
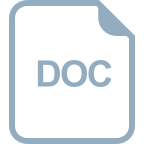
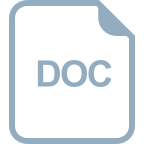