QTcpSocket使用指定端口连接服务器
时间: 2024-09-10 11:16:59 浏览: 49
QTcpSocket是Qt库中的一个类,用于处理TCP网络通信。如果你想要使用QTcpSocket来连接到特定端口的服务器,你可以按照以下步骤操作:
1. 创建QTcpSocket实例:
```cpp
QTcpSocket *socket = new QTcpSocket(this);
```
这里`this`通常是指代包含它的对象,如QObject。
2. 连接到服务器:
```cpp
QHostAddress serverAddress; // 设置服务器地址
serverAddress.setHostAddress("192.168.0.1"); // 使用IP地址替换
serverAddress.setPort(8080); // 指定你要连接的端口号
if (!socket->connectToHost(serverAddress)) {
qDebug() << "Failed to connect: " + socket->errorString();
} else {
qDebug() << "Connected to server";
}
```
这里的`connectToHost()`函数尝试连接到指定的服务器地址和端口。如果连接成功,它会返回true;否则,将打印错误信息。
3. 断开连接(当不再需要时):
```cpp
socket->close();
delete socket;
```
相关问题
QTcpSocket指定客户端端口连接服务器
在Qt库中,如果你想要使用`QTcpSocket`类从客户端连接到服务器,并指定特定的客户端端口,你可以按照以下步骤操作:
1. 首先,创建一个`QTcpSocket`对象实例:
```cpp
QTcpSocket *clientSocket = new QTcpSocket(this);
```
这里,`this`通常是指向包含这个socket对象的QMainWindow或其他QObject。
2. 连接前确保网络套接字已初始化并设置正确的主机名和端口号:
```cpp
if (!clientSocket->isValid()) {
qDebug() << "Socket not initialized.";
return; // 处理错误
}
QString hostName("your_server_ip");
quint16 port(8080); // 或者你想使用的任意端口号
connect(clientSocket, &QTcpSocket::connected,
clientSocket, &QTcpSocket::write);
connect(clientSocket, &QTcpSocket::disconnected,
this, &YourClass::onDisconnected);
clientSocket->setHost(hostName);
clientSocket->setPort(port);
```
注意替换`your_server_ip`为实际的服务器IP地址。
3. 确保连接成功后开始通信:
```cpp
if (clientSocket->connectToHost(hostName, port)) {
qDebug() << "Connected to server at " << hostName << ":" << port;
QByteArray dataToSend("Your message here"); // 要发送的数据
clientSocket->write(dataToSend);
} else {
qWarning() << "Failed to connect to the server";
}
```
4. 当连接断开时,可以处理相关的事件:
```cpp
void YourClass::onDisconnected() {
qDebug() << "Connection to server lost.";
delete clientSocket;
}
```
记得处理可能出现的异常和错误情况,例如超时、连接失败等。同时,在实际应用中,可能还需要添加适当的错误重试机制。
QTcpSocket指定使用某个端口连接服务器
在Qt框架中,如果你想要创建一个`QTcpSocket`实例并指定连接到特定的服务器端口,你可以按照以下步骤操作:
1. 首先,确保已经包含了`QTcpSocket`头文件:
```cpp
#include <QTcpSocket>
```
2. 创建一个`QTcpSocket`对象:
```cpp
QTcpSocket socket;
```
3. 设置目标地址和端口号,这通常是在程序开始或者在连接之前完成的:
```cpp
QHostAddress serverAddress; // 如果是IPv4
serverAddress.setIPv4Address(YOUR_SERVER_IP); // 将YOUR_SERVER_IP替换为你想连接的实际IP地址
int serverPort = YOUR_SERVER_PORT; // 将YOUR_SERVER_PORT替换为你想连接的具体端口号
if (!socket.connectToHost(serverAddress, serverPort)) {
qDebug() << "Failed to connect to server: " << socket.errorString();
} else {
qDebug() << "Connected to server successfully.";
}
```
这里,`connectToHost()`函数尝试连接到指定的IP地址和端口。如果连接成功,它会返回`true`,否则你会看到错误信息。
阅读全文
相关推荐
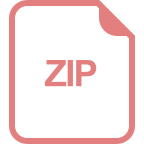
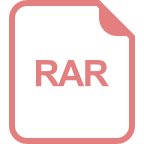
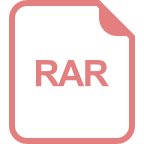
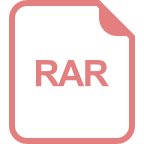
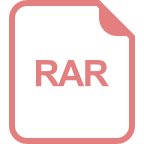
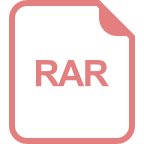
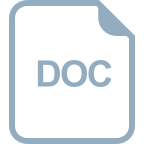









