C++读取字符串,要求大写字母,小写字母,数字,空格和其他字符数量。
时间: 2024-12-16 18:18:01 浏览: 2
在C++中,你可以使用标准库中的`std::cin`和遍历的方式来读取字符串并统计各种字符类型的数量。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <cctype> // 包含转换函数
int countCharacters(const std::string& input) {
int uppercase = 0;
int lowercase = 0;
int digits = 0;
int spaces = 0;
for (char c : input) {
if (isupper(c)) { // 判断是否为大写字母
uppercase++;
} else if (islower(c)) { // 判断是否为小写字母
lowercase++;
} else if (isdigit(c)) { // 判断是否为数字
digits++;
} else if (isspace(c)) { // 判断是否为空格
spaces++;
}
}
return {uppercase, lowercase, digits, spaces};
}
int main() {
std::string input;
std::cout << "Enter a string: ";
std::getline(std::cin, input);
auto result = countCharacters(input);
std::cout << "Uppercase letters: " << result[0] << "\n";
std::cout << "Lowercase letters: " << result[1] << "\n";
std::cout << "Digits: " << result[2] << "\n";
std::cout << "Spaces: " << result[3] << "\n";
return 0;
}
```
这个程序首先定义了四个计数器变量分别用于大写字母、小写字母、数字和空格。然后遍历输入的字符串,使用`isupper()`、`islower()`、`isdigit()`和`isspace()`等`<cctype>`库中的函数判断每个字符的类型,并相应地增加计数。
阅读全文
相关推荐
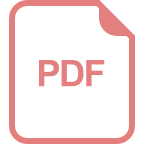
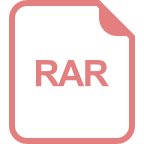
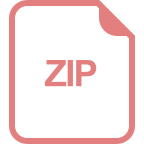















