C++读取字符串,要求大写字母,小写字母,数字,空格,其他字符共5类的数量。
时间: 2024-12-16 13:18:04 浏览: 4
在C++中,你可以使用`std::string` 类型来读取字符串,并通过遍历和条件判断来统计不同类别字符的数量。以下是一个简单的示例:
```cpp
#include <iostream>
#include <cctype> // 包含ASCII码转换函数
int countCategories(const std::string& str) {
int upperCaseCount = 0;
int lowerCaseCount = 0;
int digitCount = 0;
int spaceCount = 0;
int otherCount = 0;
for (char c : str) {
if (isupper(c)) { // 判断是否为大写字母
upperCaseCount++;
} else if (islower(c)) { // 判断是否为小写字母
lowerCaseCount++;
} else if (isdigit(c)) { // 判断是否为数字
digitCount++;
} else if (isspace(c)) { // 判断是否为空格
spaceCount++;
} else { // 其他字符(包括标点、特殊字符等)
otherCount++;
}
}
return std::make_tuple(upperCaseCount, lowerCaseCount, digitCount, spaceCount, otherCount);
}
int main() {
std::string input;
std::cout << "Enter a string: ";
getline(std::cin, input);
auto categoryCounts = countCategories(input);
std::cout << "Uppercase: " << get<0>(categoryCounts) << ", Lowercase: " << get<1>(categoryCounts)
<< ", Digits: " << get<2>(categoryCounts) << ", Spaces: " << get<3>(categoryCounts)
<< ", Other: " << get<4>(categoryCounts) << std::endl;
return 0;
}
```
在这个程序中,我们首先定义了五个计数器变量,然后遍历输入字符串中的每个字符,使用`std::isupper`、`std::islower`、`std::isdigit` 和 `std::isspace` 函数检查字符的类型。如果都不是以上四种情况,就将其归类为“其他”。
阅读全文
相关推荐
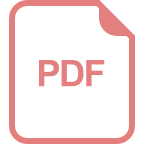
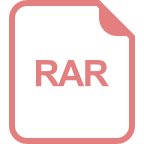
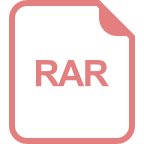















