hutool 将多个URL文件,通过流的方式压缩打包 示例代码
时间: 2024-09-12 09:13:31 浏览: 58
Hutool是一个Java工具类库,它提供了许多便利的方法来处理常见的编程任务。它可以帮助开发者通过简单的方式实现文件的压缩和打包。
以下是一个使用Hutool将多个URL指向的文件流打包压缩的示例代码:
```java
import cn.hutool.core.io.FileUtil;
import cn.hutool.core.io.IORuntimeException;
import cn.hutool.core.io.StreamProgress;
import cn.hutool.core.util.ZipUtil;
import cn.hutool.http.HttpUtil;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
public class HutoolZipExample {
public static void main(String[] args) {
try {
// 创建一个临时文件用于存储压缩后的文件
String tempZipFilePath = FileUtil.getTempPath() + "example.zip";
// 创建一个输入流集合
InputStream[] inputStreams = new InputStream[2];
String[] urls = new String[]{"http://example.com/file1.pdf", "http://example.com/file2.pdf"};
for (int i = 0; i < urls.length; i++) {
// 从URL获取文件流
URL url = new URL(urls[i]);
// 读取URL响应内容到字节数组
byte[] fileBytes = HttpUtil.downloadBytes(url);
// 将字节数组转换成输入流
inputStreams[i] = new ByteArrayInputStream(fileBytes);
}
// 使用Hutool的ZipUtil类将多个输入流压缩打包到指定路径的文件中
ZipUtil.zip(inputStreams, tempZipFilePath, null, null, (progress, percentage) -> {
// 这里可以处理压缩进度,progress是当前处理的文件名,percentage是完成的百分比
System.out.println("压缩进度: " + progress + " " + percentage + "%");
});
// 关闭所有流资源
for (InputStream in : inputStreams) {
FileUtil.close(in);
}
// 如果需要获取压缩后的文件内容,可以使用下面的方式
byte[] zipBytes = FileUtil.readBytes(tempZipFilePath);
// 做一些处理...
} catch (IORuntimeException | IOException e) {
e.printStackTrace();
}
}
}
```
请注意,上面的示例代码中,我们使用`HttpUtil.downloadBytes`方法从URL下载文件内容到字节数组,然后将这个字节数组转换为`ByteArrayInputStream`。之后我们用这个流进行压缩操作。在实际应用中,你可能需要根据实际情况来处理网络异常、IO异常等。
阅读全文
相关推荐
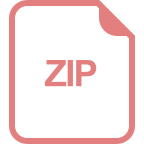
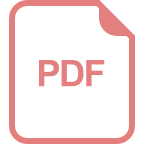
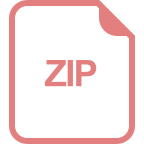















