http://192.168.5.125:5000/all-download?url=下载地址 get请求 图片地址通过这个转接再下载,原来直接下载有跨域问题
时间: 2024-11-22 12:38:27 浏览: 25
为了处理图片下载时出现的跨域问题,可以使用以下方式:
### 解决方案概述
- **目标**: 实现一个中间服务器端点来代理原始下载请求,以避免浏览器中的跨域限制。
- **方法**:
- 客户端发送 GET 请求到 `/all-download` 接口。
- 服务器接收该请求后,从提供的 URL 下载图片。
- 服务器将图片数据作为响应返回给客户端。
### 具体实现步骤
1. **前端代码调整**:
- 修改现有的下载按钮,使其调用新的代理接口。
```javascript
methods: {
// 其他方法...
downloadImage(url) {
const apiUrl = 'http://192.168.5.125:5000/all-download';
window.location.href = `${apiUrl}?url=${encodeURIComponent(url)}`;
}
}
```
2. **后端代理逻辑**:
- 在服务器上创建一个处理 `/all-download` 请求的路由。
- 使用 Node.js 和 Express 作为示例。
```javascript
const express = require('express');
const request = require('request');
const app = express();
const port = 5000;
app.get('/all-download', (req, res) => {
const imageUrl = req.query.url;
if (!imageUrl) {
return res.status(400).send('URL 参数缺失');
}
request({ url: imageUrl, encoding: null })
.on('response', function (response) {
// 设置响应头
res.set({
'Content-Type': response.headers['content-type'],
'Content-Disposition': 'attachment; filename=' + encodeURIComponent(imageUrl.split('/').pop())
});
})
.pipe(res)
.on('error', (err) => {
console.error('下载出错:', err);
res.status(500).send('下载出错');
});
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
```
### 运行和测试
1. **启动后端服务器**:
- 确保安装了 `express` 和 `request` 模块。
- 运行 `node server.js` 启动服务器。
2. **测试下载功能**:
- 打开前端应用,尝试点击“下载”按钮。
- 浏览器应通过 `/all-download` 接口发起请求,并最终下载图片。
### 总结
通过在服务器端添加一个代理接口来处理图片下载请求,可以有效地解决跨域问题。这种方式不仅简化了前端逻辑,还提高了系统的健壮性和安全性。
阅读全文
相关推荐






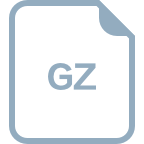








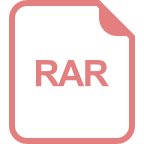
