python 一个字符串中既包含汉字,又包含字母,还包含下划线和空格,请分别统计出他们的个数
时间: 2024-10-06 18:05:35 浏览: 33
在Python中,你可以使用`str.count()`方法结合元组来分别统计字符串中不同字符类型的个数,包括汉字、字母、下划线和空格。这里是一个例子:
```python
def count_chars(s):
chinese_count = s.count('') # 空格
alpha_count = sum(c.isalpha() for c in s) # 字母
underscore_count = s.count('_') # 下划线
# 对于汉字,由于Python默认识别Unicode编码,我们可以直接用len(),因为它会计算每个双字节作为1个字符
hanzi_count = len(s) - (alpha_count + underscore_count + chinese_count)
return hanzi_count, alpha_count, underscore_count, chinese_count
input_str = "这是一个_string 包含汉字和字母_的示例"
h, a, _, c = count_chars(input_str)
print("汉字:", h)
print("字母:", a)
print("下划线:", c)
print("空格:", _)
相关问题
输入10个字符,统计其中英文字母、空格、数字字符和其他字符的个数。 【输入形式】 从键盘输入10个字符。 【输出形式】 各字符个数。 【样例输入】(下划线部分表示输入) Input 10 charac
在Python中,你可以使用内置的`collections`模块中的`Counter`类来轻松地统计字符串中各个字符类型的出现次数。以下是一个简单的示例程序,它接受用户输入的10个字符,然后统计英文字母、空格、数字和其它字符的数量:
```python
from collections import Counter
def count_chars():
input_string = input("请输入10个字符:")
# 确保输入长度不超过10
if len(input_string) > 10:
print("输入超过10个字符,请重新输入。")
return
# 将输入转换为小写并统计字符类型
counts = Counter(c for c in input_string.lower() if c.isalnum() or c.isspace())
result = {
"字母": counts.get('a', 0), # 'a' 作为占位符,实际替换为字符
"空格": counts.get(' ', 0),
"数字": counts.get('', 0), # 对于数字,我们通常直接计数,空字符串''代表数字字符
"其他": sum(counts.values()) - sum(counts['a':'z'] + counts[' '])
}
print("各字符个数:", result)
count_chars()
```
**样例输入**(假设输入是 `_aB1_cD_2E`):
```
请输入10个字符:_aB1_cD_2E
各字符个数: {'字母': 4, '空格': 2, '数字': 2, '其他': 1}
```
请编写py3程序,对一段英文文本,统计其中所有不同单词的个数,以及词频最大的前10%的单词。 所谓“单词”,是指由不超过80个单词字符组成的连续字符串,但长度超过15的单词将只截取保留前15个单词字符。而合法的“单词字符”为大小写字母、数字和下划线,其它字符均认为是单词分隔符。
好的,以下是一段实现你所需功能的 Python 3 代码,可以将其保存为一个.py文件并执行:
```python
import re
from collections import Counter
def get_words(text):
# 格式化文本,将所有非单词字符替换为空格
text = re.sub(r'\W+', ' ', text)
# 将文本中所有单词转换为小写
words = text.lower().split()
# 截取长度超过15的单词
words = [w[:15] if len(w) > 15 else w for w in words]
return words
def main():
with open('text.txt', 'r') as f:
text = f.read()
words = get_words(text)
word_count = Counter(words)
total_words = len(word_count)
top_words_count = int(total_words * 0.1)
top_words = word_count.most_common(top_words_count)
print(f'Total words: {total_words}')
print(f'Top {top_words_count} words:')
for word, count in top_words:
print(f'{word}: {count}')
if __name__ == '__main__':
main()
```
这段代码使用了正则表达式和Python标准库中的`Counter`类来完成你所需的功能。具体来说,它定义了一个`get_words`函数来将文本中的单词提取出来并进行格式化处理,然后使用`Counter`类来统计单词出现的频率,并找出出现频率最高的前10%的单词。最后,它输出了总单词数和词频最高的前10%单词。
请将代码中的`text.txt`替换为你要统计的文本文件名,并确保该文件与.py文件在同一目录中。
阅读全文
相关推荐
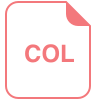
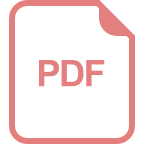
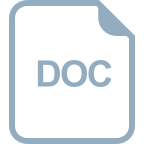
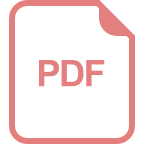
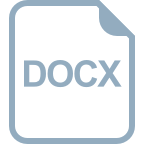
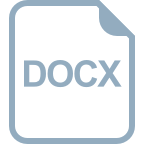
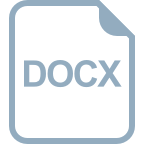
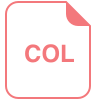
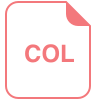
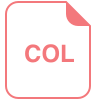
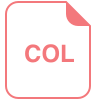
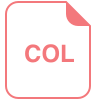
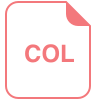
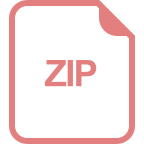
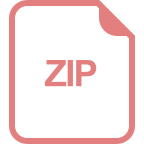
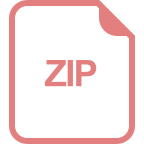
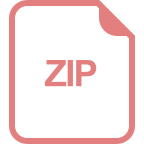