ajax ResponseEntity下载文件
时间: 2024-09-13 13:14:21 浏览: 56
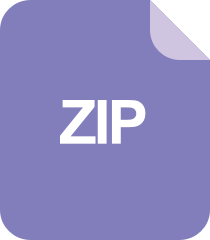
springMVC-HttpEntity(ResponseEntity)demo
AJAX (Asynchronous JavaScript and XML) 是一种用于创建异步网页应用的技术,允许前端通过JavaScript与服务器进行非阻塞的数据交换。当涉及到从服务器下载文件时,通常会利用`ResponseEntity`这个Spring MVC的概念。
在Spring框架中,`ResponseEntity`是一个泛型类型,可以包含HTTP响应的状态码、headers以及响应体内容,包括流或者是字节数组等。要使用它来下载文件,你可以这样做:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.http.ResponseEntity;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
@GetMapping("/download")
public ResponseEntity效验文件名及处理下载请求(@RequestParam("file") MultipartFile file) {
try {
// 检查文件是否有效
if (!file.isEmpty()) {
String filename = extractFileName(file);
Path targetPath = saveFileToFileSystem(filename, file);
// 创建一个Content-Disposition header,模拟浏览器下载行为
HttpHeaders headers = new HttpHeaders();
headers.setContentDispositionFormData("attachment", filename);
// 使用 ResponseEntity.ok() 和 bytes[] 或者 FileStream来设置响应内容
return ResponseEntity.ok()
.headers(headers)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(Files.readAllBytes(targetPath));
} else {
return ResponseEntity.badRequest().build(); // 返回错误状态码
}
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Error downloading file");
}
}
private String extractFileName(MultipartFile file) {
return file.getOriginalFilename();
}
private Path saveFileToFileSystem(String fileName, MultipartFile file) throws IOException {
byte[] bytes = file.getBytes();
Path filePath = Paths.get("your/download/folder/" + fileName);
Files.write(filePath, bytes);
return filePath;
}
```
在这个示例中,用户提交文件到 `/download` 路径,服务端检查文件并将其保存到本地,然后返回一个`ResponseEntity`,其中包含了文件数据和适当的HTTP头部信息以启动下载。
阅读全文
相关推荐
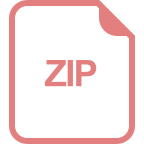
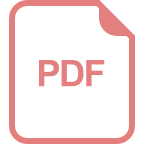



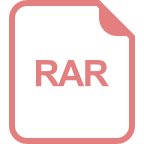
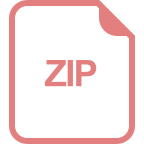
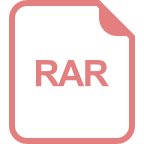
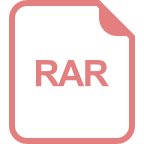
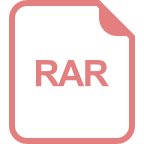
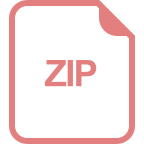
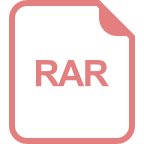
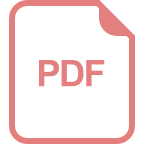
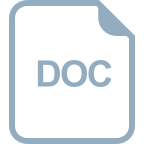
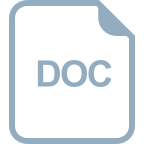


