编写一个程序exp9-2.cpp,输出在顺序表(1,2,3,4,5,6,7,8,9,10)中采用折半查找方法查找关键字9的过程)
时间: 2024-12-18 18:32:29 浏览: 16
编写一个名为 `exp9-2.cpp` 的程序,我们可以使用 C++ 来实现顺序查找(也称为线性查找),因为题目提到的是折半查找,顺序查找更适合用于简单列举的情况。这里假设顺序查找是为了展示基本过程,折半查找通常用于已排序的数据结构。
```cpp
#include <iostream>
using namespace std;
// 顺序查找函数,输入是一个整数数组和目标值,返回目标值的位置(如果找到),否则返回 -1
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; ++i) {
if (arr[i] == target) {
return i;
}
}
// 如果未找到,返回 -1 表示元素不在数组中
return -1;
}
int main() {
const int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int size = sizeof(arr) / sizeof(arr[0]);
int target = 9;
int result = linearSearch(arr, size, target);
// 输出查找过程
cout << "在有序数组 " << arr << " 中查找关键字 " << target << ":\n";
if (result != -1) {
cout << "找到了,位置是 " << result + 1 << endl;
} else {
cout << "没找到,关键字不在数组中。\n";
}
return 0;
}
```
在这个程序中,我们首先定义了一个简单的线性搜索函数 `linearSearch`,然后在主函数中创建一个有序数组并指定要查找的目标值。接着调用这个函数并打印出查找结果。注意,由于数组索引从0开始,所以在输出位置时会加上1。
阅读全文
相关推荐
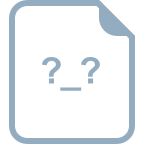
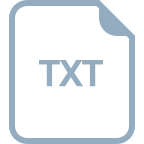
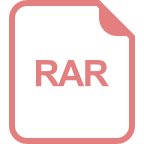
















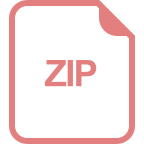