输入一串英文,统计其中每个单词出现的次数,统计结果保存在字典中以单词为key,以出现次数为value,输出统计结果(文中可包含标点,单词之间使用空格分隔)。
时间: 2024-09-21 20:04:27 浏览: 26
为了统计英文字符串中每个单词出现的次数,我们可以使用Python的内置函数和正则表达式库`re`来分割字符串,然后遍历统计。以下是实现这个功能的一个简单示例:
```python
import re
from collections import defaultdict
def count_words(input_string):
# 使用正则表达式分割字符串,只保留字母和数字组成的单词
words = re.findall(r'\b\w+\b', input_string)
# 使用defaultdict来存储单词频率,默认值为0
word_count = defaultdict(int)
# 遍历单词列表,增加计数
for word in words:
word_count[word] += 1
# 返回统计结果
return dict(word_count)
input_text = "Hello world! This is a test sentence. Hello again."
word_freq = count_words(input_text)
print("单词出现次数:", word_freq)
相关问题
请实现一个wordcount函数,统计英文字符串中每个单词出现的次数。返回一个字典,key为单词,value为对应单词出现的次数。
实现一个WordCount函数可以使用Python的内置模块`collections`中的`Counter`类,它非常适合用于计数目的场景。这是一个简单的例子:
```python
from collections import Counter
def wordcount(s):
# 将字符串转换为小写并分割成单词列表
words = s.lower().split()
# 使用Counter计算每个单词的频率
word_counts = Counter(words)
return word_counts
# 测试函数
text = "This is a test string for the wordcount function. It will count how many times each word appears."
result = wordcount(text)
print(result) # 输出:{'this': 1, 'is': 1, 'a': 1, 'test': 1, 'string': 1, 'for': 1, 'the': 1, 'wordcount': 1, 'function.': 1, 'it': 1, 'will': 1, 'count': 1, 'how': 1, 'many': 1, 'times': 1, 'each': 1, 'appears.': 1}
编写代码:从键盘输入一个英文句子或单词组成的字符串,将句子中单词以及出现的频次分别作为key和value保存在字典中,并输出
可以使用Python的字典数据结构来实现这个功能,代码如下:
```python
# 从键盘输入句子
sentence = input("请输入一个英文句子:")
# 将句子按空格分割成单词列表
words = sentence.split()
# 创建一个空的字典用于存储单词及其出现频次
word_count = {}
# 遍历单词列表,统计每个单词出现的频次
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 输出结果
print("单词\t出现频次")
for word, count in word_count.items():
print(word + "\t" + str(count))
```
当运行该程序时,会提示用户输入一个英文句子。用户输入完毕后,程序将会把句子按空格分割成单词列表,并遍历该列表统计每个单词出现的频次。最后,程序将会输出每个单词及其出现频次的字典。
阅读全文
相关推荐
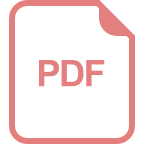
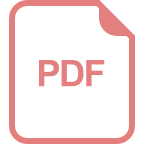
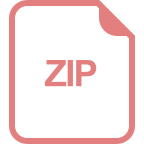













