keras模型是默认在GPU上运行吗
时间: 2024-10-14 08:16:35 浏览: 6
默认情况下,Keras会利用可用的最佳资源进行训练。如果有TPU(Tensor Processing Unit),它会优先使用TPU;如果没有TPU但有GPU,Keras会自动选择GPU进行训练;只有当TPU和GPU都不具备时,才会使用CPU[^2]。
要查看当前环境下Keras是否能够在GPU上运行,你可以使用以下方法:
1. **确认是否有GPU**:
```python
import tensorflow as tf
gpus = tf.config.list_physical_devices('GPU')
if gpus:
print("GPUs are available")
else:
print("No GPUs found")
```
2. **设置Keras使用GPU**:
如果你确定想要在GPU上训练,可以这样配置:
```python
import tensorflow.keras.backend as K
K.tensorflow_backend.set_session(tf.Session(config=tf.ConfigProto(log_device_placement=True)))
```
注意,这通常只适用于没有自动检测功能的情况。
相关问题
如何将keras模型搭载到GPU上运行
在Keras中,要让模型利用GPU运行,你需要确保以下几个步骤:
1. **确认硬件环境**[^1]:
```
import tensorflow as tf
print(tf.test.is_gpu_available()) # 如果返回True,则有可用的GPU
```
2. **设置计算设备**:
```python
gpus = tf.config.list_physical_devices('GPU')
if gpus:
try:
# 设置默认的TensorFlow会话使用GPU
tf.config.set_visible_devices(gpus[0], 'GPU')
logical_gpus = tf.config.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPU")
except RuntimeError as e:
# 即使没有物理GPU,逻辑GPU也可能存在
print(e)
```
3. **启用GPU在模型构建时**:
```python
with tf.device('/device:GPU:0'):
model = keras.Sequential([...])
```
4. **编译模型时指定优化器支持GPU**:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'], experimental_run_tf_function=False)
```
如果你已经完成了这些步骤但仍然看到CPU运算,可能是因为其他原因(如内存限制、驱动程序问题、模型配置不支持GPU)。建议检查CUDA/CuDNN版本是否匹配,以及是否有足够的显存供模型运行。
tensorflow模型怎么用gpu
要使用GPU来运行TensorFlow模型,您需要确保您的计算机上安装了适当的GPU驱动程序。然后,您需要安装TensorFlow GPU版本,它可以使用GPU来加速模型训练和推理。
安装好TensorFlow GPU版本后,您需要在代码中指定使用GPU来运行模型。您可以使用以下代码将模型放在GPU上:
```
import tensorflow as tf
# 指定使用GPU
gpu_options = tf.compat.v1.GPUOptions(per_process_gpu_memory_fraction=0.7)
sess = tf.compat.v1.Session(config=tf.compat.v1.ConfigProto(gpu_options=gpu_options))
# 定义模型
model = tf.keras.Sequential([
# ...
])
# 训练模型
model.fit(x_train, y_train, epochs=10)
```
在这个例子中,我们使用了 `tf.compat.v1.Session` 的 `config` 参数来指定了使用GPU,并且使用了 `tf.compat.v1.GPUOptions` 来设置每个GPU的内存分配比例。您可以根据自己的需要设置不同的参数。
请注意,如果您的计算机上没有GPU,您仍然可以使用CPU运行TensorFlow模型。只需使用默认的TensorFlow安装即可。
阅读全文
相关推荐
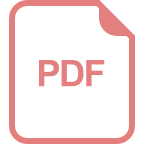
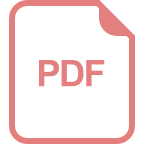
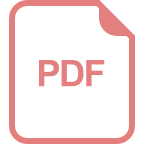













