pyqt 保存文件到指定位置
时间: 2023-11-06 16:05:28 浏览: 375
可以使用QFileDialog来实现保存文件到指定位置的功能。具体实现步骤如下:
1. 导入QFileDialog模块
```python
from PyQt5.QtWidgets import QFileDialog
```
2. 创建QFileDialog对象
```python
file_dialog = QFileDialog()
```
3. 设置文件对话框的模式和默认文件名
```python
file_dialog.setAcceptMode(QFileDialog.AcceptSave)
file_dialog.setDefaultSuffix('txt')
file_dialog.selectFile('example.txt')
```
4. 显示文件对话框并获取用户选择的文件路径
```python
if file_dialog.exec_() == QFileDialog.Accepted:
file_path = file_dialog.selectedFiles()[0]
```
完整代码示例:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建文本编辑器
self.text_edit = QTextEdit(self)
self.setCentralWidget(self.text_edit)
# 创建菜单栏
menu_bar = self.menuBar()
# 创建文件菜单
file_menu = menu_bar.addMenu('文件')
# 创建保存动作
save_action = QAction('保存', self)
save_action.triggered.connect(self.save_file)
file_menu.addAction(save_action)
def save_file(self):
# 创建文件对话框
file_dialog = QFileDialog()
file_dialog.setAcceptMode(QFileDialog.AcceptSave)
file_dialog.setDefaultSuffix('txt')
file_dialog.selectFile('example.txt')
# 显示文件对话框并获取用户选择的文件路径
if file_dialog.exec_() == QFileDialog.Accepted:
file_path = file_dialog.selectedFiles()[0]
# 将文本编辑器中的内容保存到文件中
with open(file_path, 'w') as f:
f.write(self.text_edit.toPlainText())
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
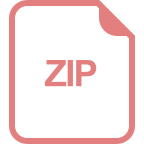
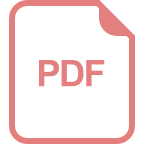















