按头和尾输入线性表各数据元素的值,建立两个链表。分别删除将两个链表中值最大和最小的结点,要求给出代码
时间: 2024-11-15 14:23:27 浏览: 0
在创建两个线性表并删除其中最大和最小节点的过程中,通常我们会定义一个链表节点结构,并用循环遍历的方式找出最大和最小值。这里是一个简单的Python示例,假设我们有一个`ListNode`类,代表链表节点:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def create_linked_list(head, values):
if not head:
head = ListNode(values[0])
for value in values[1:]:
node = ListNode(value)
node.next = head
head = node
return head
# 创建两个链表
list1_values = [5, 3, 9, 1]
head1 = create_linked_list(None, list1_values)
list2_values = [7, 1, 8, 4]
head2 = create_linked_list(None, list2_values)
# 找到每个链表的最大值和最小值节点
max1 = find_max(head1)
min1 = find_min(head1)
max2 = find_max(head2)
min2 = find_min(head2)
# 删除最大和最小节点
head1 = delete_node(head1, max1)
head1 = delete_node(head1, min1)
head2 = delete_node(head2, max2)
head2 = delete_node(head2, min2)
def find_max(head):
current = head
while current and (not max1 or current.val > max1.val):
max1 = current
current = current.next
return max1
def find_min(head):
current = head
while current and (not min1 or current.val < min1.val):
min1 = current
current = current.next
return min1
def delete_node(head, node_to_remove):
if not head:
return None
if head == node_to_remove:
return head.next
if head.next == node_to_remove:
head.next = None
return head
temp = head
while temp.next != node_to_remove:
temp = temp.next
temp.next = temp.next.next
return head
```
这个例子展示了如何创建链表、找到最大和最小节点以及删除它们。注意,这个实现假定最大值和最小值只有一个。如果存在多个相等的最大或最小值,你需要修改删除函数来处理这种情况。
阅读全文
相关推荐
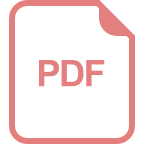
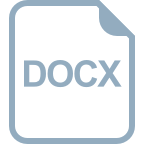
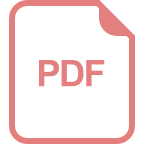


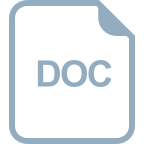












