如何编写一个 UART 串行通信的程序,以便 PC 机通过串口向单片机发送指令,实现精确控制流水灯(启动、暂停)功能?
时间: 2024-11-25 21:17:18 浏览: 9
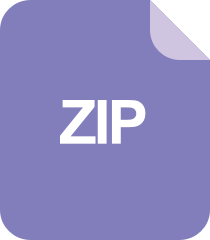
test_流水灯的控制以及串口通信_流水灯_exploreg87_

编写UART串行通信的程序通常涉及以下几个步骤,假设我们使用的是基于C语言的单片机如Arduino或使用Python的MicroPython,而PC端则可能使用像PySerial这样的库:
1. **设置硬件连接**:
- 确保单片机和PC之间的串口连接稳定,例如,单片机的TX与PC的RX相连,RX与TX相反。
2. **初始化串口**:
- 对于Arduino,可以使用`SoftwareSerial`或`HardwareSerial`类(取决于硬件支持),配置波特率(如9600)、数据位(8位)、停止位(1位)和校验位(无)。
```c
#include <SoftwareSerial.h>
SoftwareSerial uart(2, 3); // RX on pin 2, TX on pin 3
void setup() {
Serial.begin(9600); // Initialize the serial monitor for debugging
uart.begin(9600);
}
```
对于Python的MicroPython,你可以这样做:
```python
import machine
uart = machine.UART(1, baudrate=9600) # UART port 1 with 9600 baud rate
```
3. **发送指令**:
- 当需要从PC发送指令时,在PC端创建字符串,并通过`uart.write()`方法将其发送到单片机。
```c
void sendCommand(char command[]) {
int len = strlen(command);
for (int i = 0; i < len; i++) {
uart.write(command[i]);
}
uart.stop(); // Optionally pause after sending to avoid data loss
}
// On the PC side:
command = "START" or "PAUSE"
uart.write(command)
```
4. **接收并处理指令**:
- 单片机需要有一个循环来读取接收到的数据,并根据接收到的内容执行相应的操作(比如启动流水灯或暂停)。这通常涉及到一个`while`循环和`uart.read()`函数。
```c
void loop() {
if (uart.available()) {
char input = uart.read();
switch (input) {
case 'S':
// Start the LED sequence
break;
case 'P':
// Pause the LED sequence
break;
default:
// Handle unknown command or error
break;
}
}
}
```
阅读全文
相关推荐
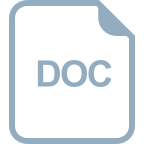
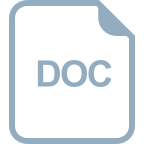
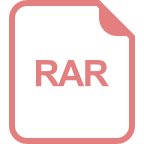
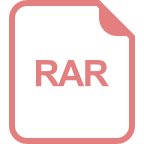
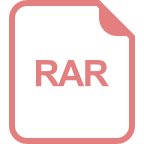
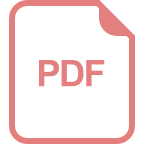
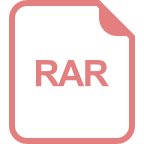
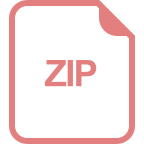
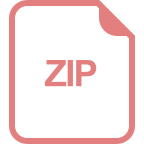
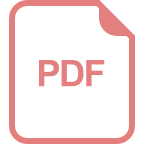
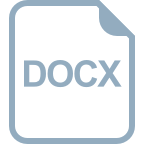
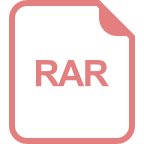
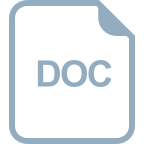
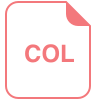



