列主元素高斯消去法c++程序代码
时间: 2024-09-26 13:00:51 浏览: 41
在C++中,列主元高斯消元法(也称为LU分解)是一种线性代数算法,用于求解线性方程组。以下是使用`std::vector`实现的一个简单的列主元高斯消元法的示例:
```cpp
#include <iostream>
#include <vector>
// 函数声明
void gauss_elimination(std::vector<std::vector<double>>& A, std::vector<std::vector<double>>& LU);
// 高斯消元核心函数
void elimination_step(const std::vector<double>& pivot_row, int pivot_index, std::vector<double>& row, std::vector<double>& L, std::vector<double>& U) {
double factor = A[pivot_index][pivot_index];
// 更新L矩阵
for (int i = 0; i <= pivot_index; ++i) {
L[i][pivot_index] /= factor;
if (i != pivot_index) {
row[i] -= A[i][pivot_index] * L[i][pivot_index];
}
}
// 更新U矩阵
for (int i = pivot_index + 1; i < A.size(); ++i) {
double temp = A[i][pivot_index];
A[i][pivot_index] /= factor;
row[i] -= temp;
U[i][pivot_index] /= factor;
for (int j = pivot_index + 1; j < A[i].size(); ++j) {
U[i][j] -= A[i][pivot_index] * U[j][pivot_index];
}
}
}
// 主函数
int main() {
std::vector<std::vector<double>> matrix(4, std::vector<double>(4)); // 4x4 矩阵示例
// 填充矩阵...
gauss_elimination(matrix, matrix); // 将A复制到LU分解后的结果
return 0;
}
// 主函数里的gauss_elimination函数
void gauss_elimination(std::vector<std::vector<double>>& A, std::vector<std::vector<double>>& LU) {
int n = A.size();
for (int k = 0; k < n; ++k) {
int pivot_row = k;
for (int i = k + 1; i < n; ++i) {
if (fabs(A[i][k]) > fabs(A[pivot_row][k])) {
pivot_row = i;
}
}
if (pivot_row != k) { // 如果发现非主元素交换行
std::swap(A[k], A[pivot_row]);
std::swap(LU[k], LU[pivot_row]);
}
elimination_step(A[k], k, A[k+1], LU[k], LU[k+1]); // 消元过程
}
// 输出L和U矩阵...
}
```
这个代码实现了高斯消元的基本步骤,并创建了两个新的矩阵`L`(上三角矩阵)和`U`(对角线元素为1的上三角矩阵),表示原矩阵的LU分解。
阅读全文
相关推荐
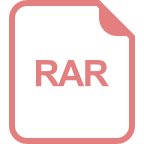
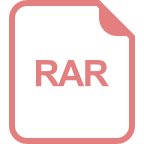
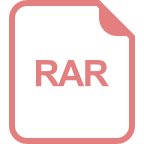

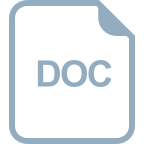
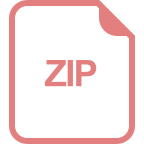
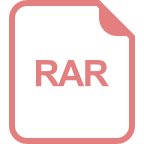
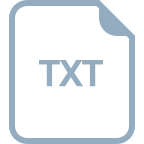
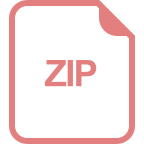
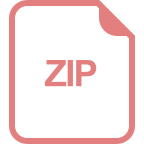

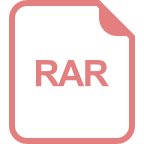
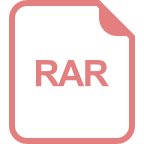
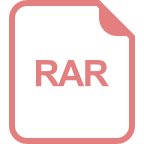
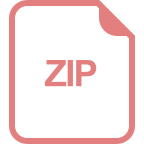
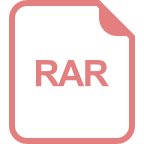
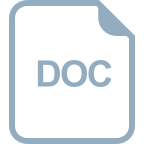
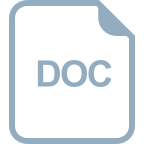
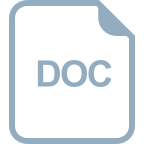