springboot netty mqtt
时间: 2024-12-26 07:17:28 浏览: 0
### 集成Netty和MQTT到Spring Boot项目
在构建基于Spring Boot的应用程序时,如果目标是实现高效的消息通信或是开发物联网(IoT)解决方案,则可以考虑通过集成Netty与MQTT协议来达成这一目的。下面提供了一种可能的方式来进行这种集成。
#### 添加依赖项
首先,在`pom.xml`文件中加入必要的Maven依赖:
```xml
<dependencies>
<!-- Spring Boot Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- Netty Server Dependency -->
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.72.Final</version>
</dependency>
<!-- Eclipse Paho MQTT Client Library -->
<dependency>
<groupId>org.eclipse.paho</groupId>
<artifactId>org.eclipse.paho.client.mqttv3</artifactId>
<version>1.2.5</version>
</dependency>
<!-- Optional: If using Spring Integration for MQTT -->
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-mqtt</artifactId>
<version>${spring-integration.version}</version>
</dependency>
</dependencies>
```
#### 创建MQTT配置类
接着创建一个用于管理MQTT连接设置的Java配置类:
```java
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
@Component
public class MqttConfig {
private static final String BROKER_URL = "tcp://localhost:1883";
private static final String CLIENT_ID = "test-client";
@Bean
public MqttClient mqttClient() throws Exception {
MemoryPersistence persistence = new MemoryPersistence();
MqttClient client = new MqttClient(BROKER_URL, CLIENT_ID, persistence);
MqttConnectOptions connOpts = new MqttConnectOptions();
connOpts.setCleanSession(true);
client.connect(connOpts);
return client;
}
}
```
此代码片段展示了如何初始化并建立至MQTT代理服务器的客户端连接[^1]。
#### 实现消息监听器服务
定义一个简单的服务组件用来处理接收到的信息:
```java
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.MqttCallback;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MessageListenerService implements MqttCallback {
@Autowired
private MqttClient mqttClient;
@Override
public void connectionLost(Throwable cause) {
System.out.println("Connection lost!");
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
System.out.printf("Received message [%s]: %s%n", topic, new String(message.getPayload()));
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
System.out.println("Delivery complete.");
}
}
```
上述实现了基本的消息接收逻辑,并将其绑定到了特定的主题上。
#### 启动应用程序
最后一步是在启动过程中订阅所需主题以及发送测试消息给指定的目标地址。这通常会在主应用入口处完成,即带有`@SpringBootApplication`注解的地方。
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
ConfigurableApplicationContext context = SpringApplication.run(Application.class, args);
try {
MqttClient mqttClient = context.getBean(MqttClient.class);
// Subscribe to topics here...
mqttClient.subscribe("/example/topic");
// Publish test messages...
MqttMessage msg = new MqttMessage("Hello from Spring Boot!".getBytes());
mqttClient.publish("/example/topic", msg);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这样就完成了整个过程——从添加所需的库直到实际运行期间的操作都已覆盖完毕。值得注意的是这里仅提供了基础框架;具体细节可能会依据个人需求有所不同。
阅读全文
相关推荐
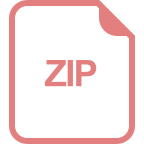
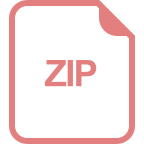
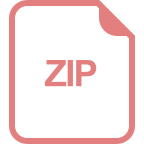
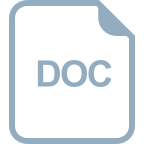
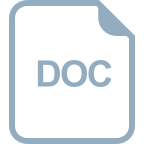

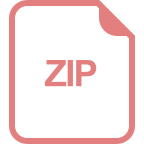
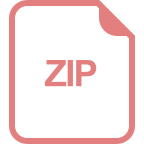
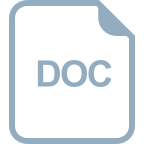
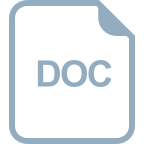
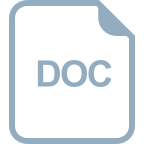
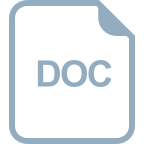
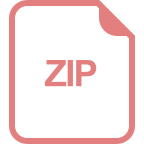
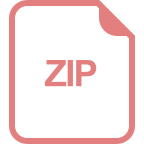
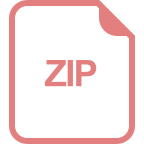
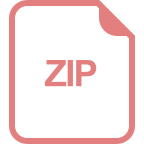
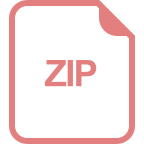
