如何用python做到这一点
时间: 2024-04-10 14:34:44 浏览: 15
要使用Python来处理Excel文件中的空值标签,你可以使用pandas库。下面是一个简单的示例代码:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('your_file.xlsx')
# 替换空值标签
df.fillna('your_value', inplace=True) # 将'your_value'替换为空值标签
# 或者删除包含空值标签的行
df.dropna(inplace=True)
# 保存修改后的文件
df.to_excel('modified_file.xlsx', index=False)
```
在这个示例中,我们使用pandas库读取Excel文件,并使用`fillna()`方法将空值标签替换为指定的值('your_value')。要删除包含空值标签的行,我们可以使用`dropna()`方法。最后,我们使用`to_excel()`方法将修改后的数据保存到一个新的Excel文件中('modified_file.xlsx')。
你可以根据你的需求自定义替换的值和保存的文件名。同时,确保在运行代码之前安装了pandas库(使用`pip install pandas`命令)。
相关问题
用python画✈️
### 回答1:
可以使用turtle库来绘制一个简单的飞机,代码示例如下:
```python
import turtle
# 创建画布
t = turtle.Turtle()
t.speed(0)
# 绘制机身
t.penup()
t.goto(-50, 0)
t.pendown()
t.setheading(0)
t.forward(100)
t.setheading(90)
t.forward(20)
t.setheading(180)
t.forward(100)
# 绘制机翼
t.penup()
t.goto(0, 20)
t.pendown()
t.setheading(-45)
t.forward(70)
t.setheading(180)
t.forward(50)
t.setheading(45)
t.forward(70)
# 绘制尾翼
t.penup()
t.goto(40, 0)
t.pendown()
t.setheading(90)
t.forward(30)
t.setheading(0)
t.forward(30)
t.setheading(-90)
t.forward(30)
# 绘制窗户
t.penup()
t.goto(-20, 10)
t.pendown()
t.dot(20)
# 隐藏画笔
t.hideturtle()
turtle.done()
```
运行代码,即可在屏幕上看到绘制的飞机。你还可以根据自己的需求修改代码,让飞机更加逼真。
### 回答2:
使用Python可以使用图像处理库来绘制一个✈️符号。这里我将使用Python的turtle库来展示如何做到这一点。
```python
import turtle
# 创建一个画布窗口和海龟对象
window = turtle.Screen()
window.bgcolor("white")
pen = turtle.Turtle()
# 设置海龟的属性
pen.speed(2)
pen.color("blue")
# 绘制飞机机身
pen.fillcolor("blue")
pen.begin_fill()
pen.forward(80)
pen.left(90)
pen.forward(20)
pen.left(45)
pen.forward(50)
pen.left(90)
pen.forward(50)
pen.left(45)
pen.forward(20)
pen.end_fill()
# 绘制飞机机翼
pen.up()
pen.left(90)
pen.forward(10)
pen.right(90)
pen.down()
pen.fillcolor("red")
pen.begin_fill()
pen.forward(100)
pen.right(120)
pen.forward(100)
pen.right(120)
pen.forward(100)
pen.end_fill()
# 绘制飞机尾翼
pen.up()
pen.right(150)
pen.forward(90)
pen.right(90)
pen.down()
pen.fillcolor("red")
pen.begin_fill()
pen.forward(30)
pen.left(120)
pen.forward(30)
pen.left(120)
pen.forward(30)
pen.end_fill()
# 隐藏海龟并打开窗口循环
pen.hideturtle()
window.mainloop()
```
通过运行上述代码,就能在Python中使用turtle库绘制一个✈️符号。这个程序会打开一个图形窗口,并在窗口中绘制一个蓝色飞机机身,红色飞机机翼和飞机尾翼。
### 回答3:
使用Python中的turtle模块可以很容易地绘制一个飞机。
首先,我们需要导入turtle模块并创建一个画布:
```python
import turtle
window = turtle.Screen()
window.bgcolor("white")
```
接下来,我们可以创建一个飞机形状的函数,并使用turtle的begin_fill()和end_fill()方法来填充颜色:
```python
def draw_plane():
turtle.fillcolor("red")
turtle.begin_fill()
turtle.seth(45)
turtle.forward(100)
turtle.circle(50, extent=180)
turtle.right(90)
turtle.forward(100)
turtle.end_fill()
```
然后,我们可以调用draw_plane()函数来绘制飞机:
```python
draw_plane()
```
最后,我们需要保持画布不关闭,以便查看绘制的飞机:
```python
turtle.done()
```
完整的代码如下:
```python
import turtle
window = turtle.Screen()
window.bgcolor("white")
def draw_plane():
turtle.fillcolor("red")
turtle.begin_fill()
turtle.seth(45)
turtle.forward(100)
turtle.circle(50, extent=180)
turtle.right(90)
turtle.forward(100)
turtle.end_fill()
draw_plane()
turtle.done()
```
运行代码后,你将看到一个以红色填充的飞机绘制在空白的白色画布上。
python第三方库使用案例
以下是一个使用第三方库的 Python 案例:
假设我们需要实现一个程序,能够从互联网上下载图片并将其转换为灰度图像。为了做到这一点,我们需要使用 Python 的第三方库 Pillow。
首先,我们需要使用 pip 命令安装 Pillow 库:
```
pip install Pillow
```
然后,我们可以编写以下代码:
```python
from PIL import Image
import requests
from io import BytesIO
# 下载图片
url = "https://www.example.com/image.jpg"
response = requests.get(url)
img = Image.open(BytesIO(response.content))
# 将图片转换为灰度图像
gray_img = img.convert('L')
# 显示灰度图像
gray_img.show()
# 保存灰度图像
gray_img.save('gray_image.jpg')
```
这个程序首先使用 requests 库从互联网上下载一张图片,并使用 BytesIO 将其转换为内存中的二进制数据。然后,使用 Pillow 库中的 Image.open() 方法将其打开并加载为一个图像对象。接下来,使用 convert() 方法将其转换为灰度图像。最后,使用 show() 方法显示灰度图像并使用 save() 方法保存为文件。
相关推荐
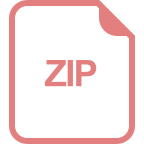
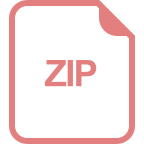
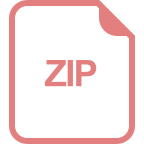












