在kylin系统中 使用.net core框架如何动态加载.so后缀的库
时间: 2024-12-01 11:20:23 浏览: 39
在Kylin系统中使用.NET Core框架动态加载`.so`(shared object)后缀的库,通常涉及跨平台运行时(比如Mono)和使用第三方工具(如P/Invoke)。由于.NET Core并不直接支持Linux上的`.so`库,以下是几个步骤来实现这一目标:
1. **使用Mono**:如果你的应用是在Linux上,或者需要支持.NET Core在非Windows平台上运行,你需要使用 Mono 运行时,它是 .NET的一个开源实现。安装相应的NuGet包,如`Mono.Posix`,它提供了对POSIX API的支持,包括动态链接。
```dotnet
dotnet add package Mono.Posix
```
2. **LoadLibrary函数**:在.NET Core中,你需要通过`DllImport`特性来引用`LoadLibrary`或`dlopen`这样的函数,它们允许你在运行时动态加载共享库。
```csharp
[DllImport("libdl", SetLastError = true)]
private static extern IntPtr dlopen(string fileName, int flags);
```
3. **GetProcAddress函数**:接着,你可以使用`GetProcAddress`函数来获取库中的函数地址,然后就可以像调本地函数一样调用它了。
```csharp
[DllImport("libdl", SetLastError = true)]
private static extern IntPtr dlsym(IntPtr handle, string symbolName);
```
4. **示例代码**:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("libdl", SetLastError = true)]
private static extern IntPtr dlopen(string fileName, int flags);
[DllImport("libdl", SetLastError = true)]
private static extern IntPtr dlsym(IntPtr handle, string symbolName);
static void Main(string[] args)
{
string libFilePath = "/path/to/your/so/library.so";
IntPtr handle = dlopen(libFilePath, 0); // RTLD_NOW | RTLD_LAZY
if (handle == IntPtr.Zero)
{
Console.WriteLine("Failed to load the shared library.");
return;
}
try
{
IntPtr funcPtr = dlsym(handle, "YourFunctionName"); // 替换为你想要调用的函数名
if (funcPtr == IntPtr.Zero)
{
Console.WriteLine("Failed to find function in the library.");
}
else
{
// 现在你可以使用Marshal.PtrToStructure将函数指针转换为所需类型的函数
Func<int> yourFunction = Marshal.GetDelegateForFunctionPointer(funcPtr, typeof(Func<int>));
int result = yourFunction(); // 调用你的函数
}
}
finally
{
dlclose(handle); // 关闭动态链接库
}
}
}
```
请注意,实际过程可能因库的具体结构和函数签名而异。对于复杂的库,你可能还需要处理异常、线程安全性和错误检查等问题。
阅读全文
相关推荐

















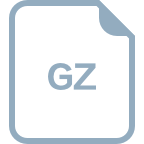