如何在C++中使用面向对象的方法设计一个学籍管理系统,实现学生信息的添加、查找和数据存储功能?请提供相关的类设计和实现代码。
时间: 2024-11-09 09:15:54 浏览: 23
在设计一个学籍管理系统时,我们首先需要定义几个核心的类,如Student类用于存储学生信息,FileSystem类用于管理文件存储,以及管理系统类ManagementSystem,它将整合Student和FileSystem类来实现用户交互和功能实现。以下是实现这些功能的详细步骤和代码示例:
参考资源链接:[C++面向对象程序设计:学生学籍管理系统设计与实现](https://wenku.csdn.net/doc/7jkqqe0v6n?spm=1055.2569.3001.10343)
首先,定义一个Student类来表示学生的数据模型。这个类可能包含私有成员变量,例如学号、姓名、性别、籍贯、成绩等,并提供公有的访问器和修改器方法:
```cpp
class Student {
private:
int studentID;
string name;
string gender;
string origin;
int mathScore;
int englishScore;
int computerScore;
public:
Student(int id, string n, string g, string o, int m, int e, int c);
void setStudentInfo(int id, string n, string g, string o, int m, int e, int c);
void displayStudentInfo() const;
// 其他方法,例如查找学生信息等
};
```
接下来,我们可以定义一个FileSystem类来处理数据的文件存储:
```cpp
class FileSystem {
public:
void saveStudentToFile(const Student& student);
Student loadStudentFromFile(int studentID);
// 其他文件管理方法
};
```
最后,我们创建一个ManagementSystem类,它将使用Student和FileSystem类来提供用户接口:
```cpp
class ManagementSystem {
private:
FileSystem fs;
vector<Student> students;
public:
void addStudent();
void findStudent();
void saveData();
void loadData();
// 其他管理系统方法
};
```
在ManagementSystem类中,addStudent方法负责添加新的学生信息,findStudent方法支持按学号或姓名查找学生,saveData和loadData方法分别用于数据的保存和加载。
具体实现代码将涉及C++文件流的操作以及异常处理,确保数据的正确读写和程序的健壮性。此外,设计时应考虑数据结构的选择,例如使用std::vector存储学生信息,以便快速访问和管理。
上述设计和代码为学籍管理系统的面向对象实现提供了基础框架。进一步的,可利用UML工具绘制类图,以更直观地描述系统设计。在项目开发过程中,通过分步实现和测试每个功能,可以逐步完善系统。最终,系统的构建将帮助学生深入理解面向对象的设计思想,同时提升编程和软件开发的实践能力。
参考资源链接:[C++面向对象程序设计:学生学籍管理系统设计与实现](https://wenku.csdn.net/doc/7jkqqe0v6n?spm=1055.2569.3001.10343)
阅读全文
相关推荐





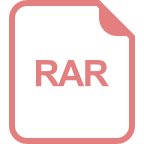
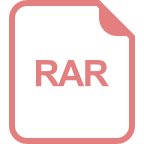
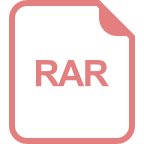










