用android代码打开蓝牙设备
时间: 2024-12-19 21:25:08 浏览: 5
在Android中,你可以通过`BluetoothAdapter`类来管理蓝牙功能。以下是基本步骤,展示如何检查蓝牙是否开启并搜索可用的蓝牙设备:
```java
// 引入所需的包
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
public class BluetoothManager {
private static final String TAG = "BluetoothManager";
// 获取蓝牙适配器实例
private BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
public void openBluetooth() {
if (mBluetoothAdapter != null) {
// 检查蓝牙是否已启用
if (!mBluetoothAdapter.isEnabled()) {
try {
// 如果未启用,请求用户启用蓝牙
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} catch (ActivityNotFoundException e) {
Log.d(TAG, "Bluetooth not available");
}
} else {
// 蓝牙已启用,开始扫描设备
startScan();
}
} else {
Log.d(TAG, "No Bluetooth adapter found");
}
}
private void startScan() {
if (mBluetoothAdapter != null && mBluetoothAdapter.isDiscovering()) {
Log.d(TAG, "Scanning already in progress...");
} else {
mBluetoothAdapter.startDiscovery(new Runnable() {
@Override
public void run() {
Log.d(TAG, "Discovering started...");
}
});
}
}
// 这是一个模拟的 onActivityResult 方法,实际应用需要处理结果
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_ENABLE_BT) {
if (resultCode == Activity.RESULT_OK) {
Log.d(TAG, "Bluetooth enabled successfully");
startScan();
} else {
Log.e(TAG, "Failed to enable Bluetooth");
}
}
}
// 示例中的REQUEST_ENABLE_BT是一个常量,用于标记请求码
private static final int REQUEST_ENABLE_BT = 0x0001;
}
```
在这个例子中,我们首先获取蓝牙适配器,然后检查它是否启用。如果未启用,我们会尝试请求用户允许蓝牙开启。一旦蓝牙开启,我们开始扫描周围的蓝牙设备。
阅读全文
相关推荐












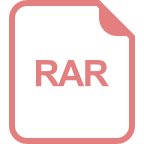



