数据结构循环队列实现舞伴配对
时间: 2024-10-26 08:16:56 浏览: 27
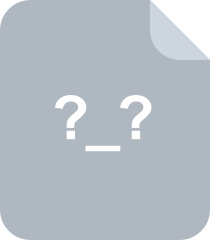
【数据结构实验】队列的应用

数据结构中的循环队列非常适合解决舞伴配对的问题。循环队列的特点在于,当队尾满了,新的元素会被添加到队列的起始位置,形成了一个环形结构。以下是使用C++实现循环队列来模拟舞伴配对的一个简单例子:
首先,我们需要定义一个`CircularQueue`类,包含队列的基本操作,如入队`enqueue()`、出队`dequeue()`以及检查队头是否有元素`front()`:
```cpp
template <typename T>
class CircularQueue {
private:
deque<T> data;
size_t head, tail;
public:
CircularQueue(size_t capacity) : data(capacity), head(0), tail(0) {}
// 插入元素到队尾
void enqueue(T value) {
if (tail == data.size() - 1) {
data.push_front(value);
tail = 0;
} else {
data.push_back(value);
tail++;
}
}
// 删除并返回队头元素(如果存在)
T dequeue() {
if (head == tail) {
return T{}; // 空队列
}
T value = data[head];
data.erase(data.begin() + head);
head = (head + 1) % data.size();
return value;
}
bool isEmpty() const { return head == tail; }
bool isFull() const { return tail == (head + 1) % data.size(); }
// 获取队头元素(如果没有,返回默认值,如-1)
T front() const {
if (!isEmpty()) {
return data[head];
}
return T{-1};
}
};
```
接着,你可以创建两个`CircularQueue`实例,分别代表男士和女士队伍,然后按照描述的过程进行操作:
```cpp
CircularQueue<int> menQueue(capacity);
CircularQueue<int> womenQueue(capacity);
// ... (其他操作如入队、匹配舞伴等)
// 检查并输出等待配对者
if (menQueue.front() != -1) {
cout << "等待配对的男士: " << menQueue.front() << endl;
} else if (womenQueue.front() != -1) {
cout << "等待配对的女士: " << womenQueue.front() << endl;
}
```
阅读全文
相关推荐
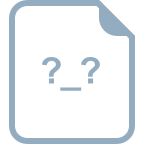
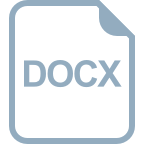


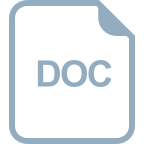
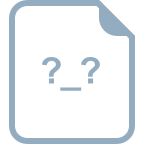
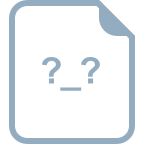
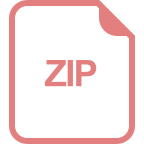
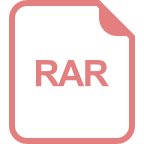
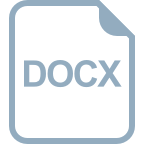
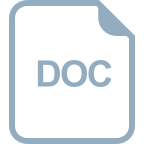
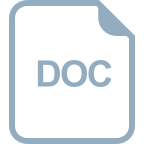
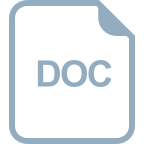
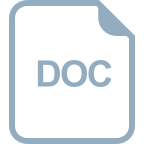
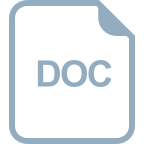

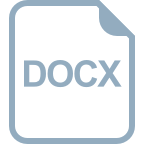
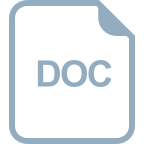