四子棋ai算法python
时间: 2023-08-25 22:15:26 浏览: 37
四子棋AI算法与五子棋算法类似,只是棋盘大小和连成胜利的条件不同。以下是一个简单的四子棋AI算法的Python实现示例:
```python
import numpy as np
# 棋盘大小
BOARD_SIZE = 7
# 棋型评估表
SCORE_TABLE = [
[10000, 1000, 1000, 10000],
[1000, 100, 100, 1000],
[1000, 100, 100, 1000],
[10000, 1000, 1000, 10000]
]
# 判断某位置是否越界
def is_out_of_range(x, y):
return x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE
# 判断某位置是否为空
def is_empty(board, x, y):
return board[x][y] == 0
# 判断某位置是否为自己的棋子
def is_own_piece(board, x, y, player):
return board[x][y] == player
# 判断某位置是否为对手的棋子
def is_opponent_piece(board, x, y, player):
return is_own_piece(board, x, y, -player)
# 判断某位置是否是胜利状态
def is_win(board, x, y, player):
directions = [(1, 0), (0, 1), (1, 1), (1, -1)] # 横、竖、斜、反斜四个方向
for dx, dy in directions:
count = 1
tx, ty = x, y
while True:
tx += dx
ty += dy
if is_out_of_range(tx, ty) or is_opponent_piece(board, tx, ty, player):
break
count += 1
tx, ty = x, y
while True:
tx -= dx
ty -= dy
if is_out_of_range(tx, ty) or is_opponent_piece(board, tx, ty, player):
break
count += 1
if count >= 4:
return True
return False
# 评估棋盘得分
def evaluate(board, player):
score = 0
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if is_own_piece(board, i, j, player):
score += SCORE_TABLE[i][j]
elif is_opponent_piece(board, i, j, player):
score -= SCORE_TABLE[i][j]
return score
# 极大极小搜索算法
def minimax(board, depth, max_depth, player):
if depth == max_depth:
return evaluate(board, player)
best_score = float('-inf') if player == 1 else float('inf')
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if is_empty(board, i, j):
board[i][j] = player
score = minimax(board, depth + 1, max_depth, -player)
board[i][j] = 0
best_score = max(score, best_score) if player == 1 else min(score, best_score)
return best_score
# AI下棋
def ai_move(board):
best_score = float('-inf')
best_move = None
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if is_empty(board, i, j):
board[i][j] = 1
score = minimax(board, 0, 3, -1)
board[i][j] = 0
if score > best_score:
best_score = score
best_move = (i, j)
return best_move
# 初始化棋盘
board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
# AI先手下棋
move = ai_move(board)
board[move[0]][move[1]] = 1
# 打印棋盘
print(board)
```
这是一个简单的极大极小搜索算法的实现,搜索深度为3。你可以根据需求调整搜索深度和评估函数来提高AI的水平。
相关推荐
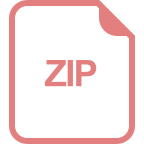
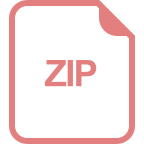
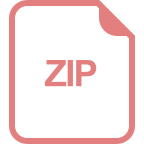














