使用C++完成但不能使用STL库
时间: 2024-09-18 07:12:36 浏览: 23
如果你需要使用C++但是禁止使用标准模板库(STL),那么代码通常会涉及到手动管理内存、数组和基本的数据结构。例如,如果你要创建一个简单链表的实现,而不依赖于`std::list`:
```cpp
#include <iostream>
// 链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 手动添加元素到链表尾部
void appendToList(ListNode*& head, int value) {
ListNode* newNode = new ListNode(value);
if (head == NULL) {
head = newNode;
} else {
ListNode* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != NULL) {
std::cout << temp->val << " ";
temp = temp->next;
}
std::cout << std::endl;
}
int main() {
ListNode* head = NULL;
// 添加元素
appendToList(head, 1);
appendToList(head, 2);
appendToList(head, 3);
// 打印链表
printList(head); // 输出: 1 2 3
return 0;
}
```
在这个例子中,我们手动实现了链表的基本操作,如节点的构造、添加新节点和打印链表。虽然这样做降低了代码的复用性和简洁性,但在某些限制环境中可能是必要的。
相关推荐
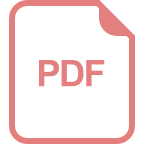
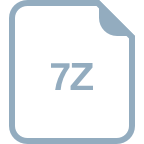
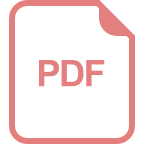














