``` const selectedRows: { name: Array<number> }[] = getSelectRows(); ```请将name的类型改为string
时间: 2024-09-25 18:16:58 浏览: 31
在 TypeScript 中,如果你想要将 `selectedRows` 数组中 `name` 字段的类型从 `number` 改为 `string`,你可以这样做:
```typescript
const selectedRows: { name: string[] }[] = getSelectRows(); // 修改了 name 的类型为 string
```
这里我们简单地把数组元素的 `name` 属性的类型从 `Array<number>` 更改为 `Array<string>`,这样每个对象的 `name` 就应该包含字符串值。
如果你想确保在调用 `getSelectRows()` 时就得到正确的类型,你还可以添加类型断言或使用泛型(如果这是接口的一部分):
```typescript
// 使用类型断言
const selectedRows = getSelectRows() as { name: string[] }[];
// 或者在定义 getSelectRows 函数时使用泛型
function getSelectRows<T extends object>(argName?: T): T[] {
const result: T[] = ...; // 实现逻辑并返回结果,假设它返回的是字符串类型的 name
return result;
}
```
阅读全文
相关推荐
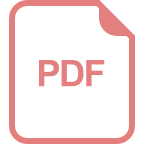
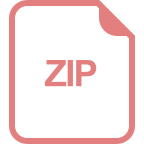
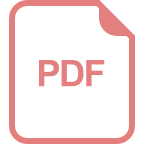
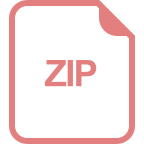
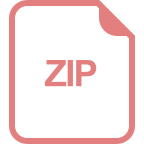
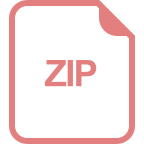
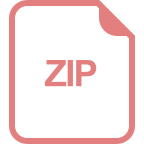
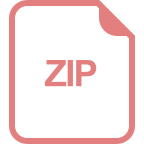
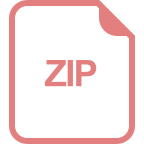
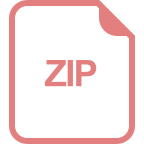
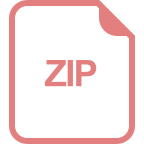
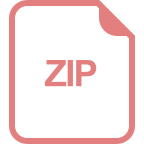
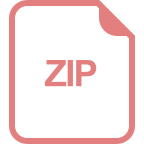
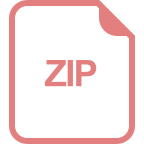
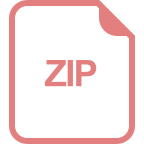
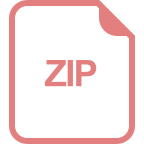
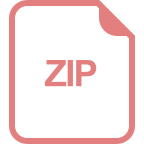
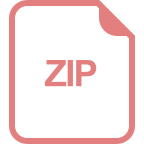
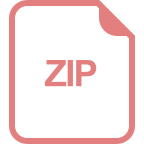