c++读写二进制文件
时间: 2024-09-25 09:21:10 浏览: 36
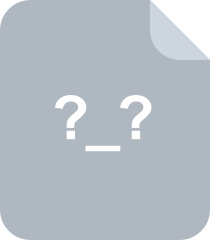
C++二进制文件读写

在 C++ 中,读写二进制文件通常涉及到 `fstream` 类库。以下是基本的操作步骤:
### 读取二进制文件
```cpp
#include <fstream>
#include <iostream>
int main() {
std::ifstream file("binary_file.bin", std::ios::binary); // 使用二进制模式打开文件
if (!file) { // 检查文件是否成功打开
std::cerr << "Failed to open the file.\n";
return 1;
}
// 定义要读取的数据类型(例如整数、浮点数等)
int data;
// 读取数据
file.read(reinterpret_cast<char*>(&data), sizeof(data));
if (file.gcount() != sizeof(data)) { // 检查读取了多少字节
std::cerr << "Failed to read the entire data.\n";
} else {
std::cout << "Read: " << data << "\n";
}
file.close(); // 关闭文件
return 0;
}
```
### 写入二进制文件
```cpp
#include <fstream>
#include <iostream>
int main() {
std::ofstream file("binary_file.bin", std::ios::binary); // 使用二进制模式创建文件
if (!file) {
std::cerr << "Failed to create the file.\n";
return 1;
}
// 准备要写入的数据(例如整数、浮点数等)
int data_to_write = 42;
// 写入数据
file.write(reinterpret_cast<char*>(&data_to_write), sizeof(data_to_write));
if (file.fail()) { // 检查写入是否成功
std::cerr << "Failed to write the data.\n";
} else {
std::cout << "Wrote: " << data_to_write << "\n";
}
file.close();
return 0;
}
```
阅读全文
相关推荐
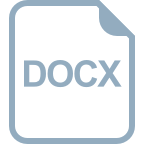
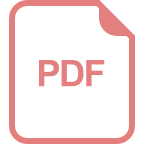

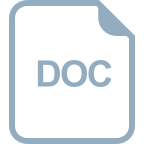

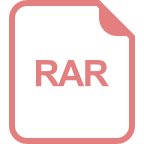
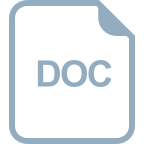
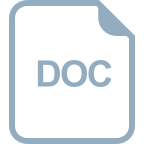
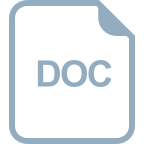
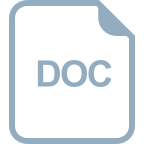
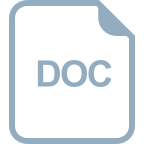
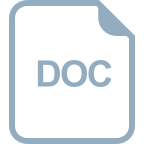
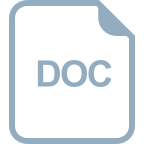
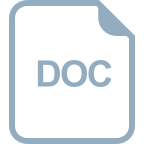



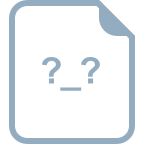