pta输入n个朋友的信息,包括姓名、生日、电话号码,本题要求编写程序,按照年龄从大到小的顺序依次输出通讯录。题目保证所有人的生日均不相同
时间: 2024-12-27 17:17:35 浏览: 9
### PTA编程实现按年龄排序的通讯录
为了实现按照年龄降序排列的朋友通讯录,可以采用多种编程语言来完成此任务。这里提供 Python 和 C++ 的解决方案。
#### 使用 Python 实现
Python 提供了简洁的数据处理方式以及内置函数 `sorted()` 来简化排序操作:
```python
from datetime import date, datetime
class Friend:
def __init__(self, name, birthday_str, phone):
self.name = name
self.birthday = datetime.strptime(birthday_str, "%Y-%m-%d").date()
self.phone = phone
@property
def age(self):
today = date.today()
return (today.year - self.birthday.year -
((today.month, today.day) < (self.birthday.month, self.birthday.day)))
def main():
friends_list = []
n = int(input())
for _ in range(n):
name, birthday, phone = input().split(',')
friend = Friend(name.strip(), birthday.strip(), phone.strip())
friends_list.append(friend)
sorted_friends = sorted(friends_list, key=lambda f: f.age, reverse=True)
for friend in sorted_friends:
print(f"{friend.name}, {friend.age} years old, Tel:{friend.phone}")
if __name__ == "__main__":
main()
```
这段代码定义了一个名为 `Friend` 的类用于存储每位朋友的信息,并计算其当前年龄;通过读取标准输入获取数据并创建对象列表后对其进行排序最后打印结果[^1]。
#### 使用 C++ 实现
对于C++, 可以利用结构体(structure)和STL中的vector容器与sort算法:
```cpp
#include <iostream>
#include <string>
#include <sstream>
#include <algorithm>
#include <iomanip>
struct Person {
std::string name;
struct tm birth_date{}; // Use this structure to store dates.
std::string tel;
bool operator<(const Person& other)const{
return mktime(const_cast<tm*>(&birth_date)) > mktime(const_cast<tm*>(&other.birth_date));
}
};
void parseDate(std::istringstream &iss, struct tm *bday){
char ch;
iss >> bday->tm_year >> ch >> bday->tm_mon >> ch >> bday->tm_mday;
bday->tm_year -= 1900; // Years since 1900
bday->tm_mon--; // Months are counted from zero
}
int main(){
size_t numPeople;
std::cin >> numPeople;
std::getchar(); // Consume newline character after number of people.
std::vector<Person> persons(numPeople);
for(auto &person : persons){
getline(std::cin, person.name, ',');
std::string tempBirthDayString;
getline(std::cin, tempBirthDayString, ',');
std::istringstream ss(tempBirthDayString);
parseDate(ss,&person.birth_date);
getline(std::cin,person.tel);
}
stable_sort(persons.begin(),persons.end());
for(const auto &p : persons){
std::cout << p.name << ", "
<< asctime(&p.birth_date); // Note that asctime adds a trailing '\n'
std::cout << "Tel:" << p.tel;
}
return 0;
}
```
上述程序首先解析日期字符串到 `tm` 结构中以便于比较两个时间戳之间的大小关系,在此基础上实现了基于出生年月日的人群排序功能。
阅读全文
相关推荐
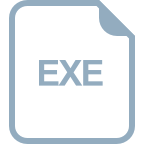
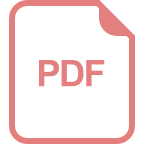
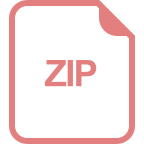















