前端传userId,查询mysql数据库中表的值中与userId相同的所有数据集合,java后端Controller,Service,Mapper接口怎么写
时间: 2024-12-23 18:15:31 浏览: 3
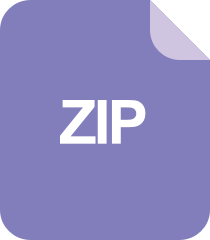
idea Java 解析json文件并导入mysql数据库
在Java的后端开发中,要实现这个功能,你需要按照MVC(Model-View-Controller)架构来组织代码。下面是大致的步骤:
**1. Controller (控制器)**
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/user/{userId}")
public User getUserData(@RequestParam("userId") Long userId) {
return userService.getUserById(userId);
}
}
```
在这个Controller里,我们定义了一个`getUserData`方法,它接受前端传来的`userId`参数,并通过@Autowired注解注入UserService。
**2. Service (服务层)**
```java
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@Autowired
private UserRepository userRepository; // 假设UserRepository是一个JPA或MyBatis的接口
@Transactional
public User getUserById(Long userId) {
return userRepository.findById(userId).orElse(null); // 使用数据库操作方法查找用户
}
}
```
在这里,`UserService`包含了业务逻辑,如从数据库获取特定ID的用户数据。`findById`方法假设是基于Spring Data JPA或者MyBatis的查询。
**3. Mapper Interface (映射器接口)**
对于JPA:
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findById(Long id);
}
```
对于MyBatis:
```java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.model.User;
public interface UserRepository extends BaseMapper<User> {
User selectByUserId(Long userId);
}
```
Mapper接口定义了如何将SQL查询转换为Java对象,JpaRepository和BaseMapper是对应ORM框架的模板方法。
阅读全文
相关推荐
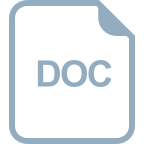
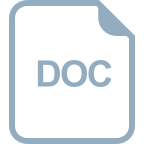


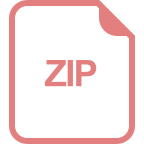
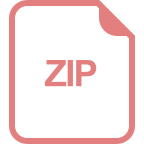
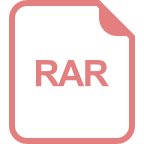
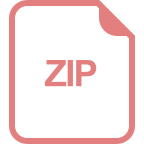
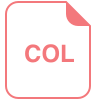








