顺序循环队列头文件验证回文
时间: 2024-10-23 22:07:28 浏览: 13
在C++中,如果你要实现顺序循环队列(Circular Queue)并验证一个字符串是否为回文,你可以这样做:
首先,我们需要创建一个`CircularQueue`类,使用数组存储数据,并处理队列满和空的情况。接着,我们可以添加一个辅助函数`isPalindrome`用于检查字符串是否是回文。
以下是一个简单的示例:
```cpp
#include <iostream>
#include <string>
class CircularQueue {
private:
std::string queue[100]; // 假设最多100个元素
int front, rear; // 队首和队尾指针
public:
// 构造函数
CircularQueue() : front(0), rear(0) {}
// 添加元素到队列尾部
void enqueue(const std::string& str) {
if ((rear + 1) % 100 == front) {
std::cout << "Queue is full.\n";
return;
}
rear = (rear + 1) % 100;
queue[rear] = str;
}
// 从队列头部移除元素
bool dequeue(std::string& removedStr) {
if (front == rear) {
std::cout << "Queue is empty.\n";
return false;
}
removedStr = queue[front];
front = (front + 1) % 100;
return true;
}
// 检查给定字符串是否为回文
bool isPalindrome(const std::string& str) const {
int start = 0, end = str.length() - 1;
while (start < end) {
if (str[start] != str[end]) {
return false;
}
start++;
end--;
}
return true;
}
};
int main() {
CircularQueue cq;
// 入队操作
cq.enqueue("madam");
cq.enqueue("level");
// 出队并检测回文
std::string removed;
if (!cq.dequeue(removed)) {
std::cout << "Dequeue failed.\n";
} else {
if (cq.isPalindrome(removed)) {
std::cout << "The dequeued string is a palindrome: " << removed << "\n";
} else {
std::cout << "The dequeued string is not a palindrome.\n";
}
}
return 0;
}
```
在这个例子中,我们首先创建了一个循环队列`CircularQueue`,然后进行入队操作(添加字符串到队列尾部),接着尝试出队并将结果传递给`isPalindrome`函数进行验证。
阅读全文
相关推荐
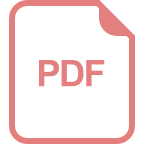
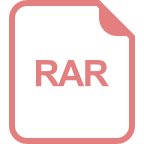
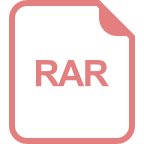
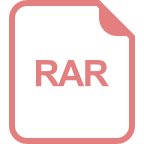
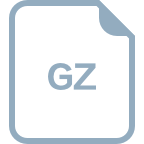
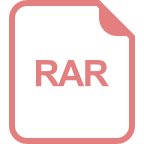
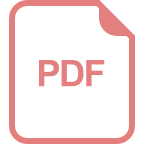
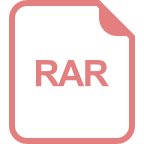
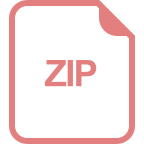
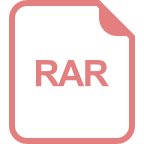