用c语言帮我实现read堵塞或者不堵塞模式,以及他返回值相应判断
时间: 2024-04-10 08:34:15 浏览: 16
以下是使用C语言实现read函数的阻塞或非阻塞模式,并对返回值进行相应判断的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <errno.h>
int set_blocking_mode(int fd, int blocking) {
int flags = fcntl(fd, F_GETFL, 0);
if (flags == -1) {
perror("fcntl");
return -1;
}
if (blocking) {
flags &= ~O_NONBLOCK;
} else {
flags |= O_NONBLOCK;
}
if (fcntl(fd, F_SETFL, flags) == -1) {
perror("fcntl");
return -1;
}
return 0;
}
int main() {
int fd;
char buffer[1024];
// 打开文件或socket等,获取文件描述符fd
// 设置为非阻塞模式
if (set_blocking_mode(fd, 0) == -1) {
return 1;
}
// 读取数据
ssize_t num_bytes = read(fd, buffer, sizeof(buffer));
if (num_bytes > 0) {
// 成功读取了一定数量的数据
printf("Read %zd bytes\n", num_bytes);
} else if (num_bytes == 0) {
// 对端关闭了连接
printf("Connection closed\n");
} else if (errno == EAGAIN || errno == EWOULDBLOCK) {
// 当前没有数据可读
printf("No data available\n");
} else {
// 出现了错误
perror("read");
return 1;
}
return 0;
}
```
在上述示例中,`set_blocking_mode`函数用于设置文件描述符的阻塞或非阻塞模式,其中`fd`为文件描述符,`blocking`为1表示设置为阻塞模式,为0表示设置为非阻塞模式。`read`函数用于从文件描述符中读取数据,返回值`num_bytes`表示实际读取的字节数。
根据返回值和`errno`的值进行相应判断:
- 如果`num_bytes`大于0,表示成功读取了一定数量的数据。
- 如果`num_bytes`等于0,表示对端关闭了连接。
- 如果`num_bytes`为-1,并且`errno`被设置为`EAGAIN`或`EWOULDBLOCK`,表示当前没有数据可读。
- 如果`num_bytes`为-1,并且`errno`被设置为其他值,表示出现了错误。可以使用`perror`函数打印出错误信息。
相关推荐
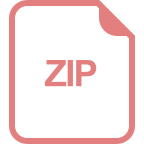
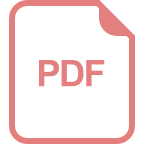
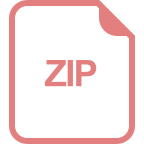














